Tutorials
Building an AI-Powered Meeting Transcript Summarizer using NodeJS and AI APIs
Learn to build an AI-driven meeting transcript summarizer with NodeJS and ApyHub API. Streamline your workflow and enhance developer productivity.
SO
Sohail Pathan
Last updated on April 24, 2024
Virtual Meetings have become an essential part of corporate collaboration, serving as the de facto way that ideas are exchanged, decisions are made, and strategies are devised. However, the true value of meetings is often diluted by their length and the complexity of the discussions. This is why note-taking and effective summaries and action points are so important. Converting long, and often pointless discussions into short (actionable) summaries, meeting attendees can quickly catch up on meetings they missed, review decisions that were made, and understand discussions without having to sift through hours and hours of recordings.
We thought it would be cool to create our summarizer. If you don't have one already keep reading. This technical tutorial will guide you through creating a simple web application that summarizes daily meeting Transcripts using ApyHub’s AI Summarize Document API using NodeJS.
Pre-requisites:
- Access to Google Meet and permission to access meeting transcripts.
- A preferred programming language environment (e.g., Python).
- ApyHub Account.
- GitHub CLI is installed on your computer.
Step 1: Clone the GitHub repository
Open your terminal, and run this command:
This command will clone the project in your local environment. Once the project is cloned, you can open the repository in the code editor.
Step 2. Install Required Packages:
Here Node Package Manager (npm), we will install all the dependencies that our application needs to summarize the meeting transcribe.
Step 3: Add your API Key to the index.js file.
Here, we will add our Secret API key from ApyHub. To generate an API key, you can visit the documentation page of AI Summarize Document API. Your secret looks like this ➡️
apy-token: APY******************************************
Copy this token and paste it in as shown in below code comment below.
Once you’ve added the API key. Save the changes using the
Ctrl+S
Key.Step 4: Running Your Application
Once you’ve added the API key. Save the changes using Ctrl+S in your terminal, and run this command.:
That’s it :) As you can see, Now you can generate a meeting transcript anytime you want :)
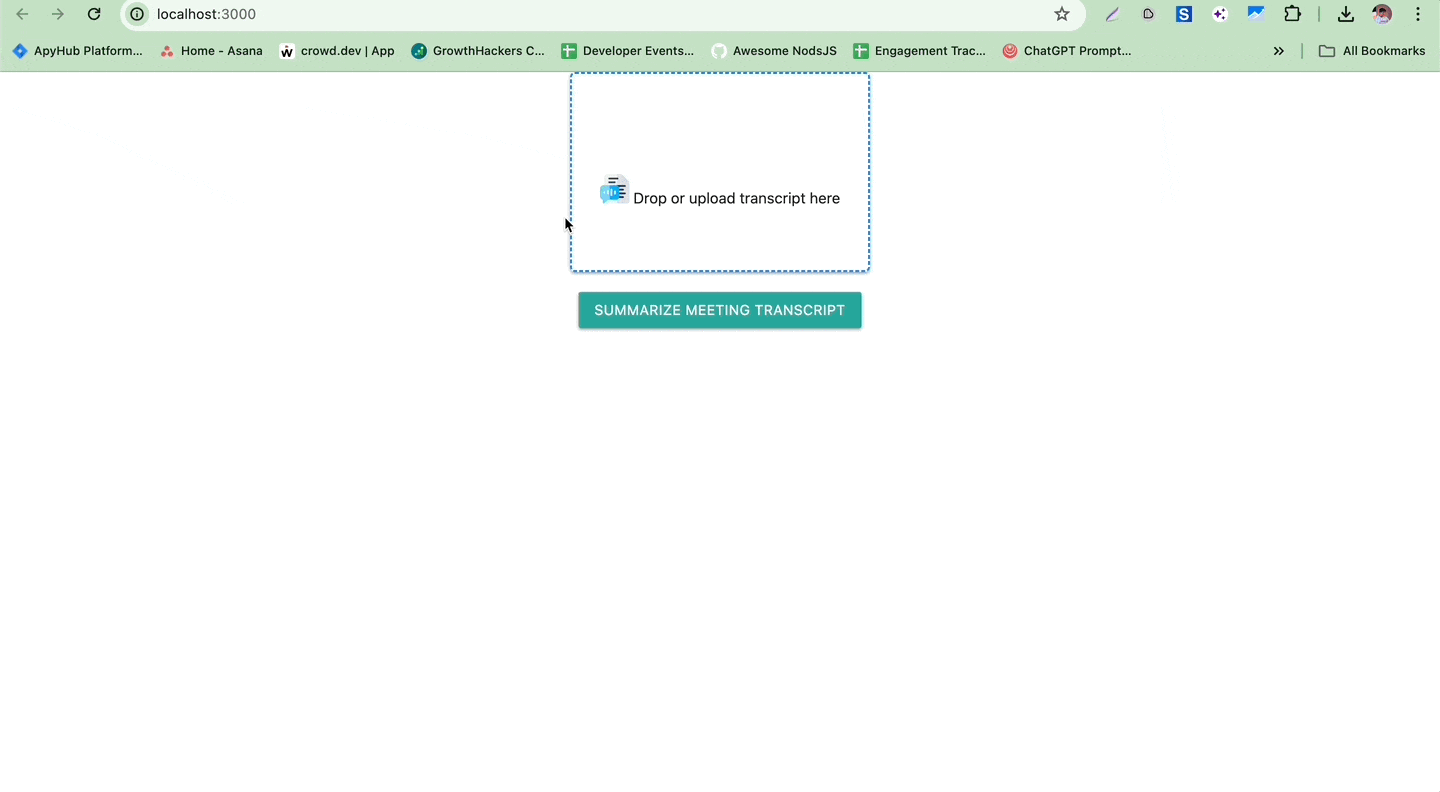
Happy with the API? We have more….
Are you a NodeJS developer? Have a look at our blog series with tutorials.