Engineering
405 Method Not Allowed: What It Means and How to Fix It Fast
Fix the 405 Method Not Allowed error with this technical guide. Learn the causes of wrong methods, server configs, API rules—and solutions.
MU
Muskan Sidana
Last updated on April 16, 2025
The 405 Method Not Allowed error hits when your HTTP request—GET, POST, PUT, DELETE—gets rejected by a server that recognizes the method but doesn’t permit it for the resource. It’s not a vague bug; it’s a precise “you’re not welcome” with an Allow header pointing to valid methods.
Want an even more streamlined API experience? ApyHub provides a powerful suite of API tools that simplify testing and automation.
What is 405 Error?
The 405 Method Not Allowed error is an HTTP status code that shows rejection. The server recognizes your method—GET, POST, PUT, DELETE, whatever—but the resource you’re hitting doesn’t support it.
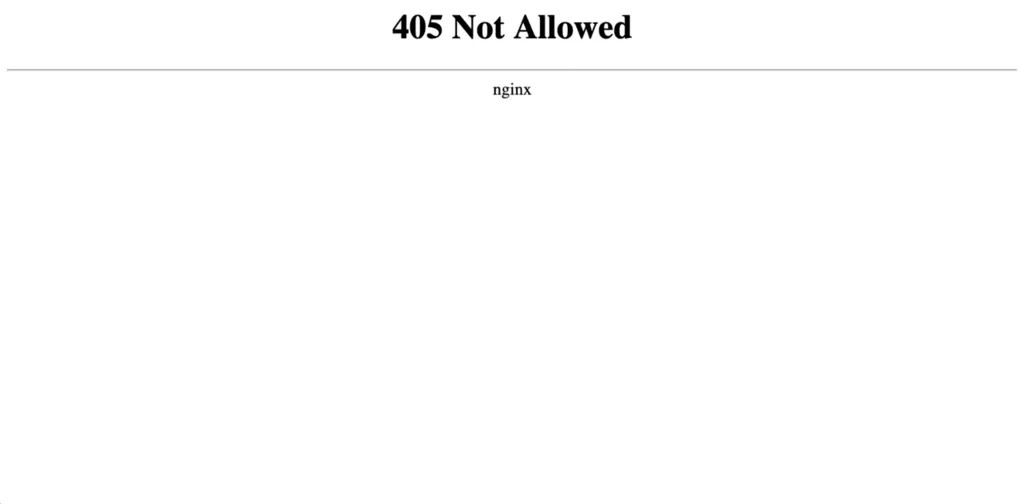
HTTP methods define the action: GET fetches, POST creates, PUT updates, DELETE removes. When your method doesn’t match the resource’s allowed list, the server responds with a 405, often tossing an
Allow
header your way to hint at what’s permitted.Here’s a quick example. You send a POST to a read-only endpoint:
POST /api/data HTTP/1.1
Host: example.com
Content-Type: application/json
{"key": "value"}
The server fires back:
HTTP/1.1 405 Method Not Allowed
Allow: GET, HEAD
Content-Type: text/html
Translation: “POST? Nope. Stick to GET or HEAD.” The challenge isn’t spotting the error—it’s nailing down why it’s blocked and fixing it fast.
How to Fix the 405 Error
Let’s get to work. Fixing a 405 Method Not Allowed error means pinpointing the cause and hammering it out. Here’s the playbook:
1. Validate the HTTP Method
Start here. Match your method to the resource’s expectations. API docs or server specs will list what’s allowed. Sending a PUT to create data? Switch to POST. Use curl to test:
curl -X GET <https://api.example.com/data> -i
If it’s a 405 with
Allow: POST
, adjust your request.2. Dig Into Server Configs
Servers like Apache or Nginx can block methods. Check the configs:
Apache: Open
.htaccess
or httpd.conf
. Look for Limit
directives:<Limit GET POST>
Order Allow,Deny
Allow from all
</Limit>
Need PUT? Add it:
<Limit GET POST PUT>
Order Allow,Deny
Allow from all
</Limit>
Nginx: Check
location
blocks in nginx.conf
: location /api {
limit_except GET POST {
deny all;
}
}
Expand it:
location /api {
limit_except GET POST DELETE {
deny all;
}
}
Restart the server after changes—
systemctl restart apache2
or nginx -s reload
.3. Confirm API Endpoint Rules
APIs are strict. If
/users
only takes POST, don’t send DELETE. Hit the docs or use OPTIONS to probe:curl -X OPTIONS <https://api.example.com/users> -i
Response might show:
HTTP/1.1 200 OK
Allow: POST, GET
Adjust your call accordingly.
4. Check Security Filters
WAFs or server rules might block methods. If a WAF’s in play, review its logs or ruleset. For Cloudflare, tweak the firewall settings to allow DELETE if it’s legit. Same goes for server-side filters—don’t blindly loosen security, but ensure valid methods pass.
5. Fix Framework Routing
Using a framework? Ensure routes support your method. In Express:
app.delete('/posts/:id',(req, res) => {
// Delete logic
});
No DELETE handler? Add it, or you’re stuck with 405.
6. Permissions Check
Even if the method’s allowed, you might lack rights. APIs often tie methods to roles. Include auth headers:
curl -X DELETE <https://api.example.com/posts/1> -H "Authorization: Bearer <token>"
No token, no access—405 or 403 could hit.
7. Parse Server Logs
Logs reveal the “why.” For Apache, check
/var/log/apache2/error.log
:[Wed Oct 25 10:00:00.2023] [error] Method PUT not allowed for /api/data
Nginx:
/var/log/nginx/error.log
:2023/10/25 10:00:00 [error] 12345#0: *1 method not allowed
Logs point to config or method issues—follow the trail.
Common Scenarios Triggering the 405 Error
This error doesn’t just happen randomly. Here’s where it rears its head in the wild:
- Wrong HTTP Method: You’re using POST when the endpoint demands PUT. REST APIs are picky—
/users
might allow POST for creation but reject PUT because it’s not built for updates at that level. - Server Config Blocks: Apache or Nginx might restrict methods. Security policies often lock down DELETE or PUT to prevent chaos, but a sloppy config can overreach and block legit methods.
- API Endpoint Rules: APIs enforce method restrictions. Hit a GET-only endpoint with a DELETE? Instant 405. Check the docs—devs often miss this.
- Security Overreach: Web Application Firewalls (WAFs) or server rules might nix methods like TRACE or OPTIONS to dodge exploits, triggering 405s even when the method’s valid.
- Framework Routing Snafus: In Express.js or Flask, if a route only handles GET and you sling a POST at it, you’re toast. No handler, no dice.
Real example: You’re managing a content API with
/posts
. It’s set up for GET and POST:// Express.js
app.get('/posts', (req, res) => res.json(posts));
app.post('/posts', (req, res) => res.status(201).json(newPost));
Send a DELETE to
/posts
? You get:HTTP/1.1 405 Method Not Allowed
Allow: GET, POST
The fix seems obvious—use the right method—but when servers, APIs, or frameworks muddy the waters, it’s not always that clean.
How Tools Handle Real-Life 405 Cases
Tools can slash troubleshooting time. Let’s compare how they tackle 405 errors in practice, not just list features.
- Postman: Fire off requests with different methods and see the
Allow
header in the response. Real case: testing a/users
endpoint. POST works, PUT fails with 405. Postman showsAllow: POST, GET
. You adjust the method instantly. Downside: no server config insights—you’re still digging manually.
cURL: Raw and fast. Hit an endpoint with
X
to test methods: curl -X PUT <https://api.example.com/data> -i
-
Get a 405? Check
Allow
and pivot. It’s lightweight but lacks automation—great for one-offs, tedious for bulk testing. -
ApyHub: Built for scale. ApyHub’s testing tools let you script method checks across endpoints. Real case: a payment API throws 405 on DELETE. ApyHub’s logs highlight the endpoint only takes POST. You fix the call in minutes. Bonus: its 130+ pre-built APIs (PDF processing, validation) mean you’re not just debugging—you’re building faster. Catch? It’s overkill for tiny projects.
Postman’s GUI shines for quick tests, cURL’s lean for CLI fans, but ApyHub bridges testing and development, cutting 405 errors off at the pass.
Conclusion
The 405 Method Not Allowed error isn’t a mystery—it’s a signal. Wrong method, bad config, or strict API? Pinpoint it with method checks, config audits, and logs. Tools like ApyHub turbocharge the fix, catching errors before they hit production. Next time a 405 stalls you, you’ve got the playbook execute it.
Want the shortcut? ApyHub’s catalog delivers 130+ battle-tested APIs. Need to process PDFs, validate data, handle payments—done. Fix 405 errors faster with built-in testing tools.
FAQ: Tackling the 405 Method Not Allowed Error
1. What exactly causes a 405 Method Not Allowed error?
The error occurs when the server understands your HTTP method (e.g., GET, POST, PUT) but the requested resource doesn’t support it. For instance, sending a DELETE to
/api/orders
built only for GET triggers a 405, often with an Allow
header like Allow: GET, POST
. To diagnose, check API documentation or use ApyHub’s endpoint testing tools to quickly identify valid methods without trial and error.2. How do I discover which HTTP methods an endpoint supports?
Use an OPTIONS request to query the endpoint:
curl -X OPTIONS <https://api.example.com/products> -i
The response includes an
Allow
header, e.g., Allow: GET, POST, HEAD
. For faster results, ApyHub’s API catalog and testing suite can automate this process, scanning multiple endpoints and listing supported methods in seconds, especially useful for large-scale APIs.3. Why would a server block specific HTTP methods?
Servers like Apache or Nginx restrict methods like PATCH or DELETE for security or due to misconfiguration. For example, an Nginx config might include:
location /api {
limit_except GET POST {
deny all;
}
}
This blocks all but GET and POST. Update configs to allow required methods, then verify with ApyHub’s debugging tools to ensure changes don’t introduce vulnerabilities while resolving 405 errors.
4. Can authentication issues lead to a 405 error?
Not directly, but insufficient permissions can mimic the issue. An API might restrict methods like DELETE to specific roles. Without proper credentials, you might hit a 405 or 403. Test with auth headers:
curl -X DELETE <https://api.example.com/orders/1> -H "Authorization: Bearer <token>" -i
ApyHub’s authentication APIs simplify token management, ensuring your requests match endpoint permissions to avoid method-related errors.
5. How can I prevent 405 errors during development?
Catch mismatches early by validating methods against API specs. Simulate requests before deploying. ApyHub excels here—its 130+ pre-built APIs include testing tools that flag method errors instantly. For example, attempting a PUT on a POST-only endpoint triggers an alert, letting you fix it pre-launch, saving debugging time.
6. What role do server logs play in fixing a 405 error?
Logs pinpoint whether the issue is a config or method mismatch. Check Nginx’s
/var/log/nginx/error.log
:2025/04/14 10:00:00 [error] 1234#0: *1 method not allowed
Or Apache’s
/var/log/apache2/error.log
:[Mon Apr 14 10:00:00.2025] [error] Method PUT not allowed for /api/data
Use these to trace restrictions, then test fixes with ApyHub’s tools to confirm the endpoint accepts your method post-update.
7. Can a Web Application Firewall (WAF) cause a 405 error?
Yes, WAFs like Cloudflare may block methods like TRACE or PATCH to prevent exploits, even if the endpoint allows them. Review WAF rules to whitelist valid methods. For example, adjust Cloudflare’s firewall to permit DELETE. ApyHub’s testing suite can simulate requests to detect if a WAF is the culprit, helping you tweak settings without compromising security.
8. How do I handle 405 errors in a framework like Express.js?
In Express, a 405 occurs if a route lacks a handler for your method. For
/items
with only GET:app.get('/items', (req, res) => res.json(items));
A POST request fails. Add the handler:
app.post('/items', (req, res) => res.status(201).json(newItem));
Test routes with ApyHub’s API tools to ensure all methods are covered before deployment, avoiding 405 surprises.