Engineering
Advanced Email Validation using ApyHub’s API in NodeJS
The tutorial provides a step-by-step guide on validating email with ApyHub DNS Email Validation API. It also covers the need for such a utility, the technical implementation, and the potential use cases of the tool.
SO
Sohail Pathan
Last updated on May 11, 2023
Email authentication has become increasingly important in recent years for the overall security of online communication, by ensuring authenticity, and protection against email fraud, phishing and other malicious activities.
Email validation can help address the issue; here are some advantages of using email validation:
- It can help detect syntax errors, typos, and invalid email addresses that can cause email delivery failures
- Network issues and over-aggressive blacklists can also result in email delivery failures, but email validation can help ensure reliable email delivery
- By implementing email validation, businesses can ensure that their email campaigns reach their intended recipients, improve their sender reputation, and maintain a positive brand image
- Additionally, email validation can help prevent potential data breaches and protect sensitive information that could be compromised through fraudulent emails
ApyHub’s Email Validation API is a cloud-based API service that allows developers to easily integrate email validation functionality into their applications. This API is designed to validate email addresses in real-time, providing accurate and reliable results that can help organisations reduce email bounce rates, prevent fraud, and improve their email marketing efforts.
How does it work?
ApyHub Email Validation API uses a multi-step process to validate email addresses. Here is an overview of how the API works:
- Syntax check: The API first checks the syntax of the email address to ensure that it conforms to the standard format. This includes checking for missing "@" or "." symbols and ensuring that the address does not contain any invalid characters.
- Standard Response: The API is a RESTful API that delivers data in JSON format.
- Domain verification: The API then verifies the email address domain by checking its DNS records. This helps ensure that the domain is valid and active and can receive email.
- Spam trap and disposable email check: Finally, the API checks the email address against known spam traps and disposable email address providers. This helps prevent fraudulent or invalid email addresses from being used. This list gets updated from a wide variety of sources daily basis to make sure you get the most up-to-date and accurate information to protect your system from potential threats related to spam and fraud.
(Looking for other ways to ensure security for your website or application? Here’s why and how you can use Secure Archives to share files)
Quick tutorial
In this tutorial, we will be going to build an authentication app using Mongoose for storing emails in a database. While the user signup, we will check whether the email is valid or not.
Prerequisites
- Node.js v16+
- An ApyHub account
- Git CLI
- To get started with ApyHub you first need to register for a free account to get an API Key (apy-token). You can use Google Authentication or GitHub Authentication as well.
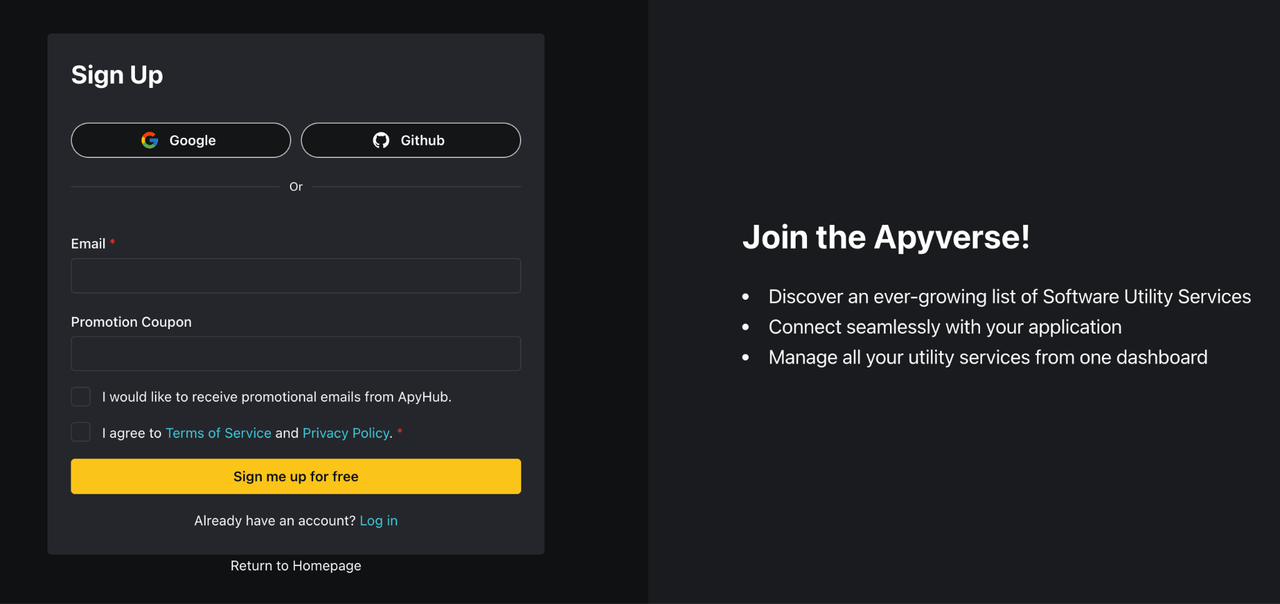
Once your signup is successful, ApyHub creates a default application with APY-TOKEN. You can find the token by navigating to Dashboard → API Keys → Default Application
Eg. APY*************************************************CZR
- Clone the starter project from the repository:
- Install dependencies
- Add your Secret APY-TOKEN in server.js
- Start the app
- Finally, Navigate to http://localhost:3000, You will able to see the Signup page.
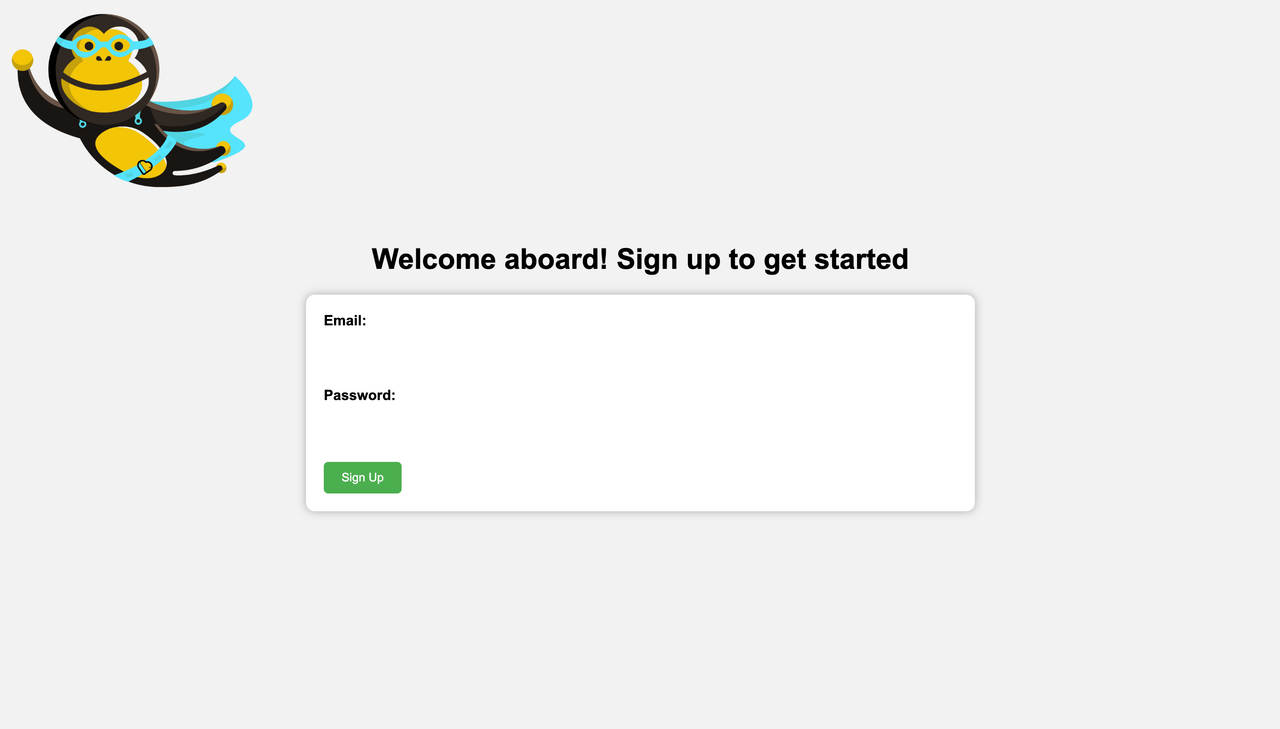
Note: The authentication module mentioned in this project is provided solely as a recommendation for the project structure. While we highly encourage its use and integration according to your architecture pattern.
Use Cases:
- Bulk email verification: Enterprises may have large email lists that need to be validated for accuracy and deliverability. An email validation API can be used to check the validity of each email address in the list, ensuring that emails are sent only to valid addresses.
- Fraud prevention: Email validation APIs can also be used to detect fraudulent email addresses used in phishing attacks, preventing unauthorised access to enterprise systems.
- Marketing campaigns: Email validation APIs can help enterprises ensure that their marketing emails reach the intended recipients by verifying the email addresses in their lists and removing invalid addresses.
- Compliance: Email validation APIs can help enterprises comply with data protection regulations by ensuring that emails are sent only to users who have provided valid consent.
- Customer support: Email validation APIs can be used to verify the email addresses of customers who submit support requests, ensuring that the enterprise is communicating with the correct customer.
So, if you're looking to improve email deliverability, reduce bounce rates and avoid spam accounts, Try out the API NOW ⬇️