Engineering
Mastering Axios GET Params: Efficient API Calls with Real-World Applications
Master Axios GET params with this detailed guide. Learn advanced techniques, optimize performance, and integrate with ApyHub APIs. Level up your JavaScript skills with real-world examples and code!
MU
Muskan Sidana
Last updated on April 15, 2025
In web development, efficiently handling HTTP requests is a cornerstone of building dynamic, responsive applications. Axios, a widely-adopted JavaScript library, simplifies this process with its promise-based architecture and intuitive API. Specifically, its handling of GET requests with parameters—referred to here as axios get params—stands out for its clarity and power.
Handling API interactions efficiently is key to building fast, reliable apps. In this guide, we'll dive into mastering Axios GET params with real-world examples you can immediately apply.
After you’re ready to level up further, we’ll also show how ApyHub’s production-ready APIs fit seamlessly into your Axios workflows.
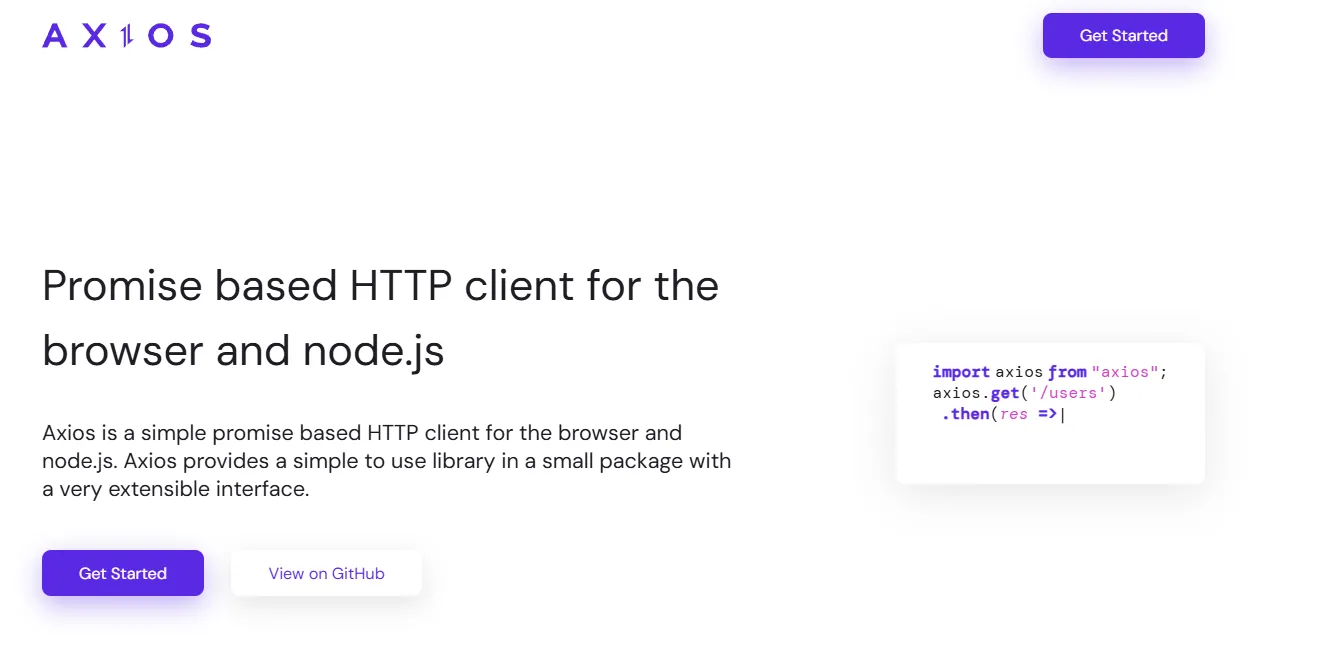
What is Axios GET Params?
When you fire off a GET request, parameters (or query parameters) are the key-value pairs tacked onto the URL to tell the server what data you want. Think
?userId=123
or ?category=tech&limit=10
. Axios makes this process seamless by letting you pass a params
object that it serializes into a query string for you. No manual string concatenation, no headache.Here’s a quick example to set the stage:
const axios \= require('axios');
async function fetchUser(userId) {
const response \= await axios.get('\<https://api.example.com/users\>', {
params: { userId }
});
return response.data;
}
fetchUser(123).then(data \=\> console.log(data)).catch(err \=\> console.error(err));
Axios takes the
params
object { userId: 123 }
and builds https://api.example.com/users?userId=123
. Clean, right? This is the foundation of axios get params, and it scales effortlessly as your needs grow.Advanced Techniques for Axios GET Params
Real-world apps don’t stop at single parameters. You’re juggling multiple params, optional values, and edge cases. Let’s break down how Axios handles these scenarios with precision.
How to Pass Multiple Query Parameters in an Axios GET Request
When your request needs more than one parameter, just stack them in the
params
object. Axios automatically manages the rest:async function searchItems(category, minPrice, maxPrice) {
const response \= await axios.get('\<https://api.example.com/items\>', {
params: {
category,
minPrice,
maxPrice
}
});
return response.data;
}
searchItems('gadgets', 50, 200).then(data \=\> console.log(data));
This generates a URL like
https://api.example.com/items?category=gadgets&minPrice=50&maxPrice=200
. Axios serializes everything, even nested objects or arrays, which we’ll tackle next.Handling Optional Parameters
Not every parameter is mandatory. Use dynamic object construction to keep your code flexible:
async function getOrders(customerId, status) {
const params \= { customerId };
if (status) params.status \= status;
const response \= await axios.get('\<https://api.example.com/orders\>', { params });
return response.data;
}
getOrders(456, 'pending').then(data \=\> console.log(data));
Here,
status
only gets added if it’s provided. The resulting URL adapts: ?customerId=456&status=pending
or just ?customerId=456
.Serializing Complex Data
What about arrays or nested objects? Axios has you covered. Pass them in
params
, and it’ll encode them properly:async function filterProducts() {
const response \= await axios.get('\<https://api.example.com/products\>', {
params: {
tags: \['tech', 'new'\],
sort: { field: 'price', order: 'asc' }
}
});
return response.data;
}
This might produce
?tags[]=tech&tags[]=new&sort[field]=price&sort[order]=asc
. You can tweak this behavior with paramsSerializer
if your API expects a different format:const response \= await axios.get('\<https://api.example.com/products\>', {
params: { tags: \['tech', 'new'\] },
paramsSerializer: params \=\> {
return Object.entries(params).map((\[key, value\]) \=\> \`${key}=${value.join(',')}\`).join('&');
}
});
Now it’s
?tags=tech,new
. Flexible and powerful.Error Handling Done Right
Errors happen—network drops, bad responses, you name it. Axios gives you granular control:
async function fetchData(endpoint, params) {
try {
const response \= await axios.get(endpoint, { params });
return response.data;
} catch (error) {
if (error.response) {
console.error(\`Server responded with ${error.response.status}:\`, error.response.data);
} else if (error.request) {
console.error('No response received:', error.request);
} else {
console.error('Request setup failed:', error.message);
}
throw error;
}
}
This catches everything from 404s to timeouts, letting you respond accordingly.
Comparing Axios to Other Tools in Real-Life Scenarios
How does Axios stack up against other options like the Fetch API or Superagent? Let’s skip the feature lists and dive into practical use cases.
Scenario 1: Filtering a Product Catalog
Imagine you’re building an e-commerce filter system. You need to fetch products with params like category, price range, and sort order.
- Axios:
async function filterProducts(filters) {
const response \= await axios.get('\<https://api.store.com/products\>', {
params: filters
});
return response.data;
}
filterProducts({ category: 'shoes', minPrice: 20, maxPrice: 100 });
Axios simplifies handling the params effortlessly and throws in JSON parsing for free.
- Fetch API:
async function filterProducts(filters) {
const url \= new URL('\<https://api.store.com/products\>');
Object.entries(filters).forEach((\[key, value\]) \=\> url.searchParams.append(key, value));
const response \= await fetch(url);
if (\!response.ok) throw new Error(\`Status: ${response.status}\`);
return response.json();
}
Fetch works, but you’re manually building the URL and handling response parsing. More code, more room for mistakes.
- Superagent:
const superagent \= require('superagent');
async function filterProducts(filters) {
const response \= await superagent.get('\<https://api.store.com/products\>').query(filters);
return response.body;
}
Superagent’s
query()
method is slick, but its ecosystem isn’t as robust as Axios’s.Verdict: Axios wins for simplicity and built-in response handling, especially when requests pile up.
Scenario 2: Polling an API with Dynamic Params
Say you’re polling a status endpoint where params change based on user input.
- Axios: Use interceptors to tweak params on the fly:
const instance \= axios.create({
baseURL: '\<https://api.status.com\>'
});
instance.interceptors.request.use(config \=\> {
config.params \= { ...config.params, timestamp: Date.now() };
return config;
});
async function pollStatus(id) {
const response \= await instance.get('/status', { params: { id } });
return response.data;
}
- Fetch: You’d need to rebuild the logic manually each time.
- Superagent: Offers chaining but lacks interceptors out of the box.
Verdict: Axios’s interceptors make it a standout for dynamic, reusable logic.
What We Like / Don’t Like About Axios GET Params
What We Like:
- Effortless Param Handling: The
params
object cuts out URL string hacks. - Flexibility: Serializes arrays, objects, and custom formats with ease.
- Interceptors: Modify requests globally without touching every call.
- Ecosystem: Huge community, tons of plugins.
What We Don’t Like:
- Size Overhead: Adds ~13KB minified—consider this for lightweight apps.
- Overkill for Simple Tasks: Fetch might suffice for one-off requests.
Optimizing Axios GET Params for Performance
Performance matters. Slow requests kill user experience. Here’s how to tune axios get params for speed.
Caching Responses
Repeated requests? Cache them. Use
axios-cache-adapter
:const { setup } \= require('axios-cache-adapter');
const api \= setup({
baseURL: '\<https://api.example.com\>',
cache: { maxAge: 10 \* 60 \* 1000 } // 10 minutes
});
async function getCachedData(params) {
const response \= await api.get('/data', { params });
return response.data;
}
This slashes server hits for identical params.
Paginating Large Datasets
Fetching 10,000 records at once is a disaster. Paginate instead:
async function getRecords(page, limit) {
const response \= await axios.get('\<https://api.example.com/records\>', {
params: { page, limit }
});
return response.data;
}
getRecords(2, 50).then(data \=\> console.log(data));
Control the data flow and keep your app snappy.
Throttling Requests
Too many requests? Throttle them with a utility:
function throttle(fn, wait) {
let lastCall \= 0;
return (...args) \=\> {
const now \= Date.now();
if (now \- lastCall \>= wait) {
lastCall \= now;
return fn(...args);
}
};
}
const throttledGet \= throttle(async params \=\> {
const response \= await axios.get('\<https://api.example.com/data\>', { params });
return response.data;
}, 1000);
throttledGet({ query: 'test' });
This caps requests to once per second.
Integrating Axios GET Params with ApyHub
ApyHub offers a treasure trove of APIs—think text analysis, image processing, and more. Pairing it with Axios is a no-brainer. Grab an API key from ApyHub, and let’s integrate.
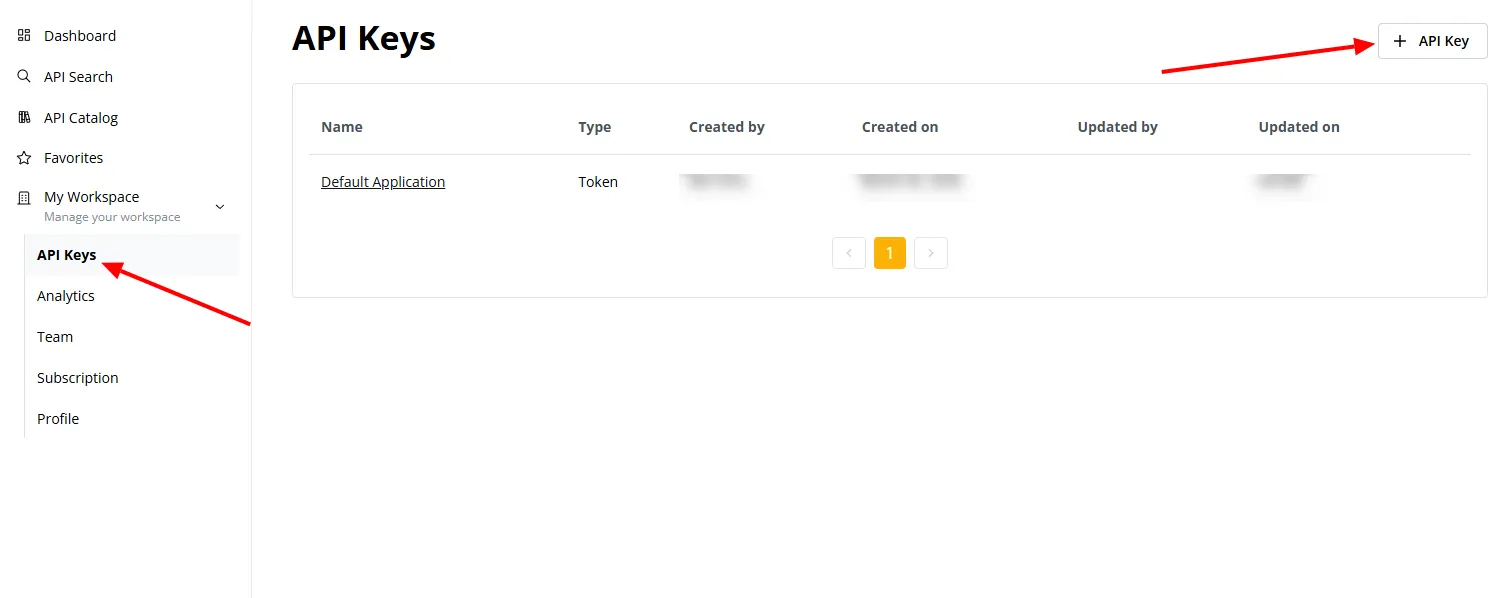
Fetching Data from ApyHub
Here’s how to query an ApyHub endpoint with axios get params:
async function analyzeText(text) {
const response \= await axios.get('\<https://api.apyhub.com/analyze/text\>', {
params: { text },
headers: { 'Authorization': 'Bearer YOUR\_API\_KEY' }
});
return response.data;
}
analyzeText('Axios is awesome\!').then(data \=\> console.log(data));
ApyHub’s API takes the
text
param and returns insights. Axios streamlines the process of the request, headers, and response parsing.Dynamic Queries with ApyHub
Need to tweak params based on user input? Easy:
async function getUserStats(userId, period) {
const response \= await axios.get('\<https://api.apyhub.com/stats/user\>', {
params: { userId, period },
headers: { 'Authorization': 'Bearer YOUR\_API\_KEY' }
});
return response.data;
}
getUserStats(789, 'monthly').then(stats \=\> console.log(stats));
This pulls user stats filtered by period—smooth and scalable.
Conclusion
Axios transforms the way you handle GET requests with parameters, blending simplicity with power. From serializing complex params to integrating with platforms like ApyHub, it’s a tool that adapts to your needs.
Ready to take your API game further? Dive into ApyHub. Its vast API library pairs perfectly with Axios, letting you build smarter, faster apps without breaking a sweat. Explore ApyHub today and see how it can turbocharge your next project.
FAQ
How do I pass parameters in an Axios GET request?
You can pass parameters in an Axios GET request using the
params
object. Axios automatically serializes it into a query string:axios.get('https://api.example.com/users', {
params: { userId: 123 }
});
This will send a request to:
https://api.example.com/users?userId=123
.Can Axios handle array or nested object parameters in GET requests?
Yes, Axios supports arrays and nested objects in GET params. For example:
params: {
tags: ['tech', 'new'],
sort: { field: 'price', order: 'asc' }
}
Axios serializes them into query strings like
tags[]=tech&tags[]=new&sort[field]=price&sort[order]=asc
. You can also customize the format using paramsSerializer
.How can I send optional GET parameters using Axios?
To send optional parameters, conditionally construct the
params
object:const params = { userId };
if (status) params.status = status;
axios.get('https://api.example.com/orders', { params });
This ensures only available parameters are included in the request URL.
What’s the difference between Axios and Fetch when passing query params?
Axios simplifies query parameter handling with its
params
option. In contrast, Fetch requires manual URL construction:// Axios
axios.get(url, { params: { category: 'tech' } });
// Fetch
const urlObj = new URL(url);
urlObj.searchParams.append('category', 'tech');
fetch(urlObj);
Axios also includes automatic JSON parsing and better error handling out-of-the-box.
How can I integrate Axios with third-party APIs like ApyHub?
You can easily integrate Axios with platforms like ApyHub by passing
params
and authentication headers:axios.get('https://api.apyhub.com/analyze/text', {
params: { text: 'Hello world' },
headers: { 'Authorization': 'Bearer YOUR_API_KEY' }
});
This makes Axios a powerful tool for interacting with external APIs efficiently.