Engineering
What is a Bearer token and how does it work?
Dive into bearer token authentication’s technical details, how it works, its advantages, and real-world use cases.
MU
Muskan Sidana
Last updated on April 17, 2025
Bearer token authentication powers secure API interactions across modern applications. It’s fast, stateless, and widely adopted. Unlike older methods, it ditches server-side session tracking for a token-based handshake.
Want to skip the API grind? Meet ApyHub. We’ve built 130+ production-ready APIs for PDFs, data validation, image magic, and more—so you don’t have to. No setup. No headaches. Just grab-and-go tools to automate the boring stuff.
Why code it yourself? Ship in minutes, not weeks.
What is Bearer Token Authentication?
Bearer token authentication secures API endpoints by passing a token in the
Authorization
header. Typically, this token is a JSON Web Token (JWT) or an OAuth access token. The client grabs it after authenticating with a server, then sends it with every request. The server validates it—signature, expiration, claims—and grants or denies access. No credentials clutter the exchange after the initial handshake.How Bearer Token Authentication Works
The flow is tight and efficient. Here’s how it breaks down:
- Client Authenticates: The client hits an authentication endpoint with credentials (e.g., username/password or client ID/secret). The server verifies and returns a token.
- Token in Flight: The client attaches the token to the
Authorization
header:Authorization: Bearer <token>
. - Server Validates: The API server checks the token’s integrity—signature, expiration, issuer. Valid? Access granted. Expired or tampered? Denied.
- Resource Access: The server processes the request, no session lookup needed.
For example, a JWT might look like this:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c
This encodes a header, payload, and signature. The server decodes it to verify authenticity.
Comparing Bearer Token Authentication to Alternatives
Bearer tokens aren’t the only game in town. Let’s stack them up:
Basic Authentication
Basic auth sends
username:password
in Base64 per request. It’s dead simple:Authorization: Basic dXNlcjpwYXNz
But it’s weak—credentials travel every time, and Base64 isn’t encryption. Bearer tokens send a signed token once, validated server-side. More secure, less chatty.
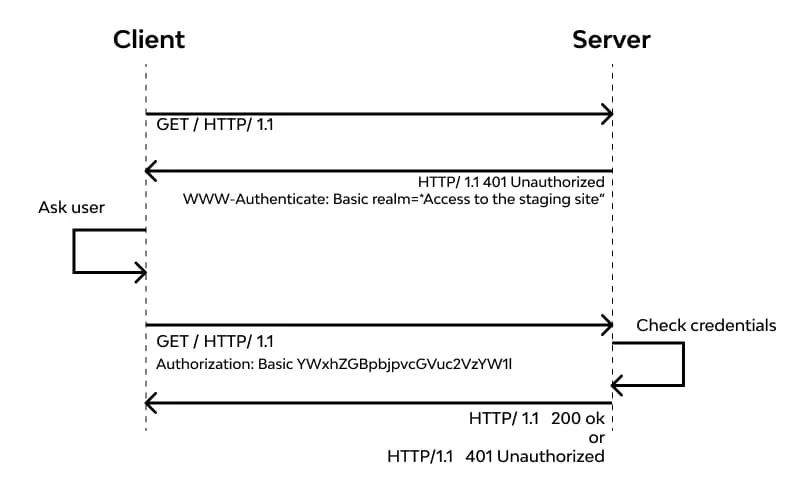
API Keys
API keys are static strings:
X-API-Key: abc123xyz
They’re lightweight but lack expiration or revocation. Bearer tokens, especially JWTs, embed metadata (e.g.,
"exp"
, "sub"
) and rotate via refresh tokens. ApyHub’s APIs favor bearer tokens for this dynamism.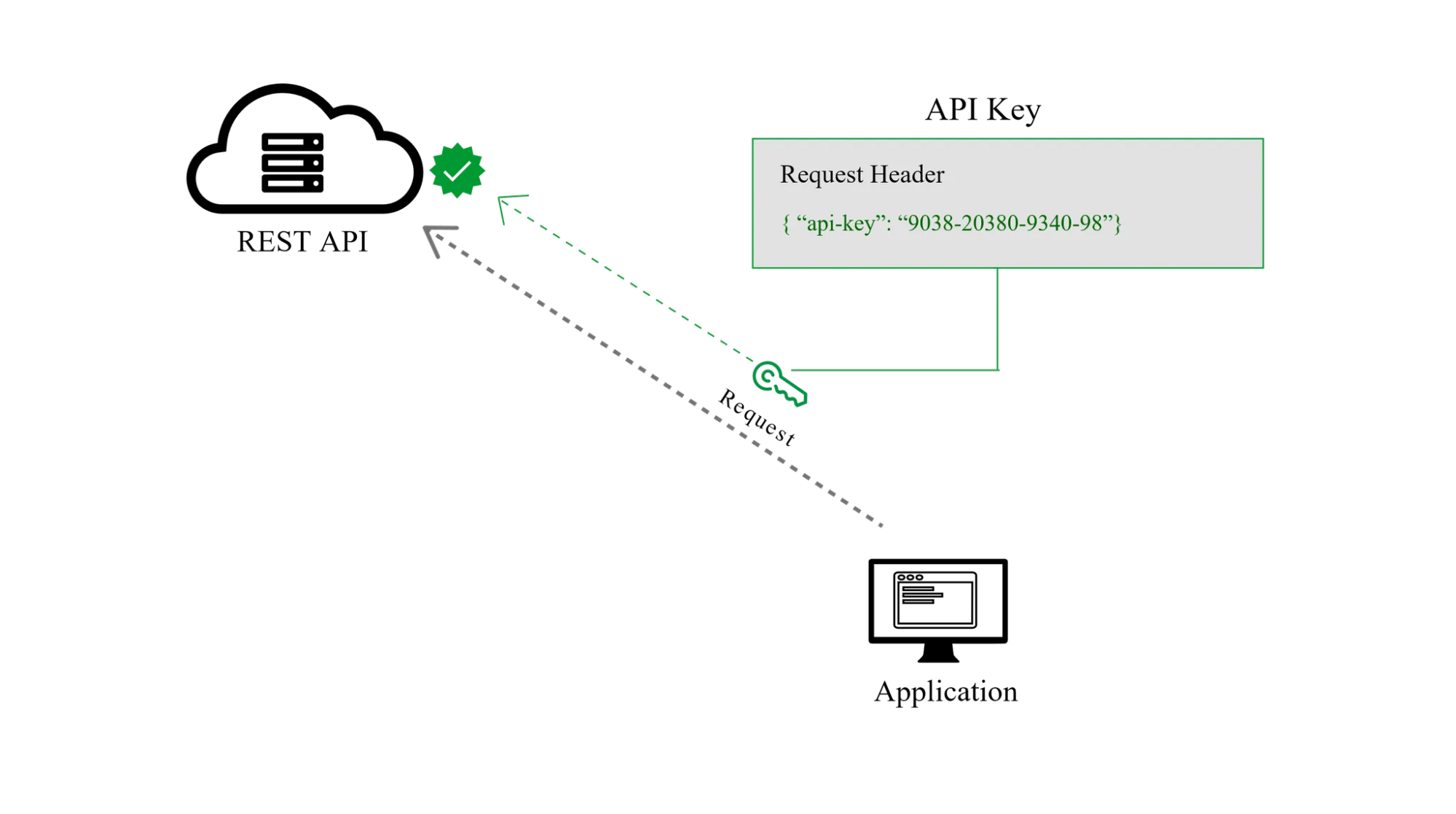
OAuth 2.0
OAuth 2.0 often uses bearer tokens but adds authorization layers—scopes, consent flows. It’s heavier:
GET /resource HTTP/1.1
Host: api.example.com
Authorization: Bearer abc123
Host: api.example.com
Authorization: Bearer abc123
Bearer tokens alone skip OAuth’s complexity for simpler use cases. ApyHub balances this—its APIs use bearer tokens but sidestep OAuth overhead unless needed.
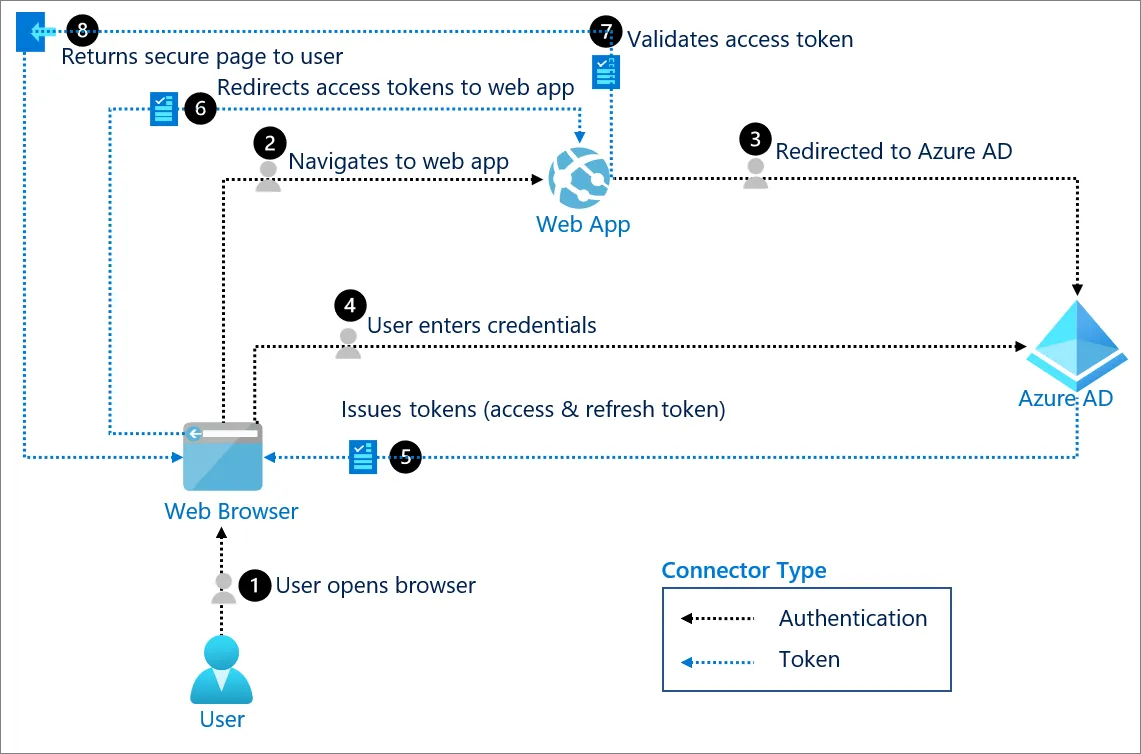
Implementing Bearer Token Authentication
Let’s get hands-on. Here’s how to wield bearer tokens in code.
Python with requests
import requests
# Grab the token
auth_url = "<https://auth.example.com/token>"
payload = {"username": "user", "password": "secret"}
response = requests.post(auth_url, json=payload)
token = response.json()["access_token"]
# Hit a protected endpoint
api_url = "<https://api.example.com/data>"
headers = {"Authorization": f"Bearer {token}"}
response = requests.get(api_url, headers=headers)
print(response.json() if response.status_code == 200 else "Access denied")
The server issues a token (e.g., JWT), and you reuse it until it expires.
Node.js with axios
const axios = require("axios");
async function getData() {
// Authenticate
const auth = await axios.post("<https://auth.example.com/token>", {
username: "user",
password: "secret",
});
const token = auth.data.access_token;
// Fetch data
const response = await axios.get("<https://api.example.com/data>", {
headers: { Authorization: `Bearer ${token}` },
});
console.log(response.data);
}
getData().catch((err) => console.error(err));
Same flow—fetch token, send it, profit.
ApyHub Integration
ApyHub’s APIs expect a bearer token in the same header. Here’s a twist—it's dashboard generates tokens for you:
To create a new API Token in ApyHub,
From your dashboard, click on API Keys on the left panel. In the API Keys page, click on the + API Keys button.
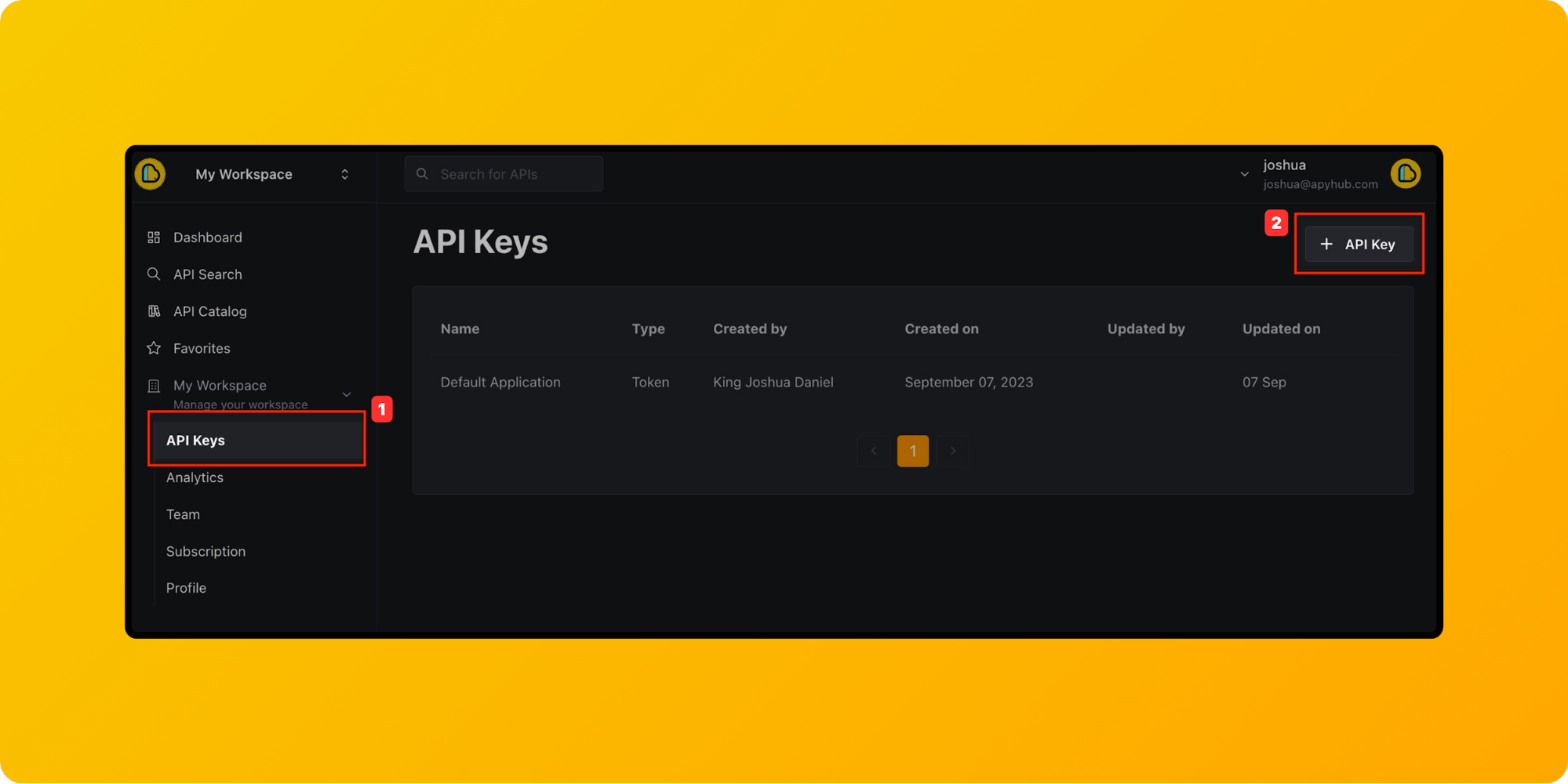
Select Authentication type as Token, give a token name, and click onthe Create button.
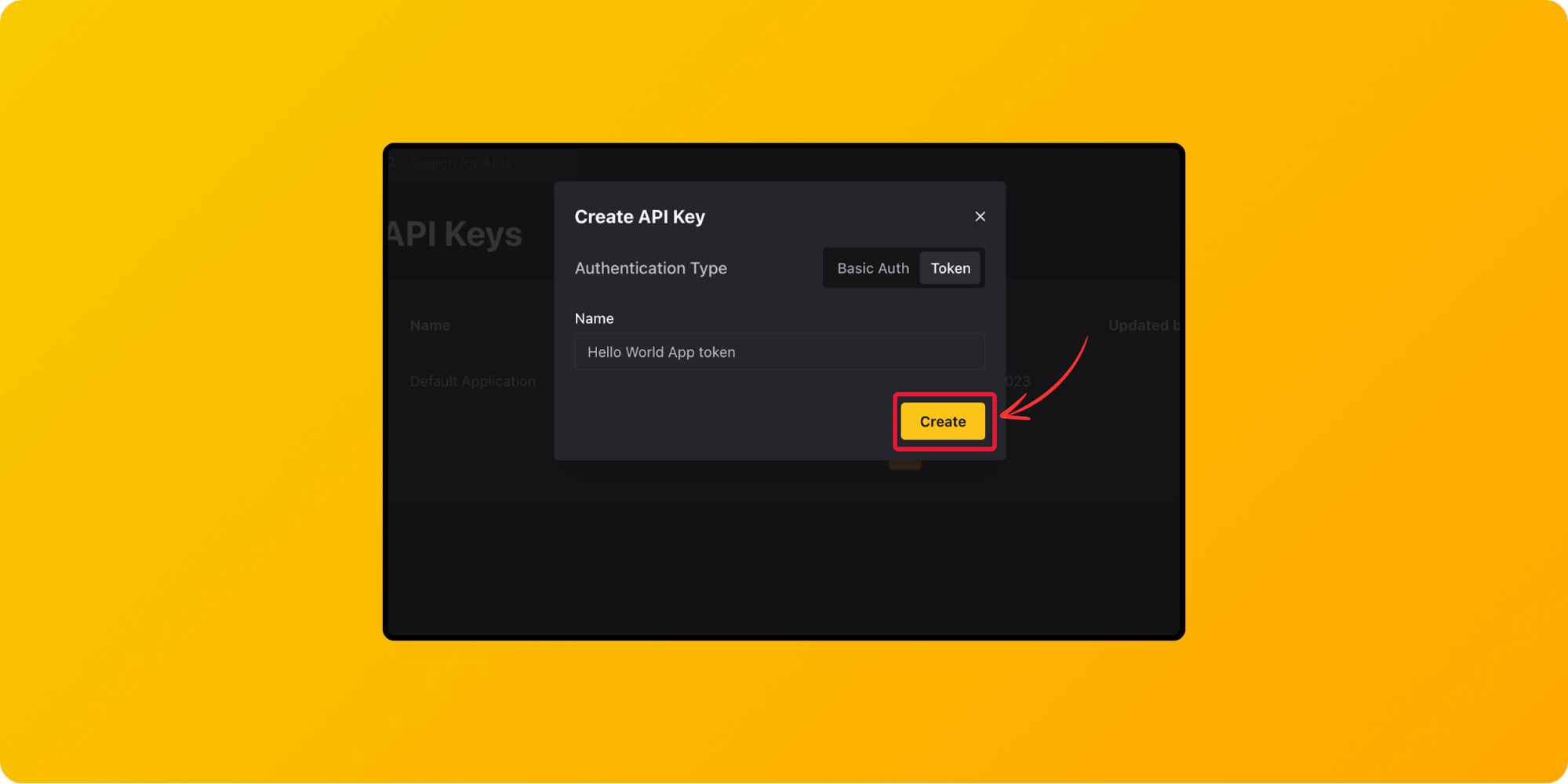
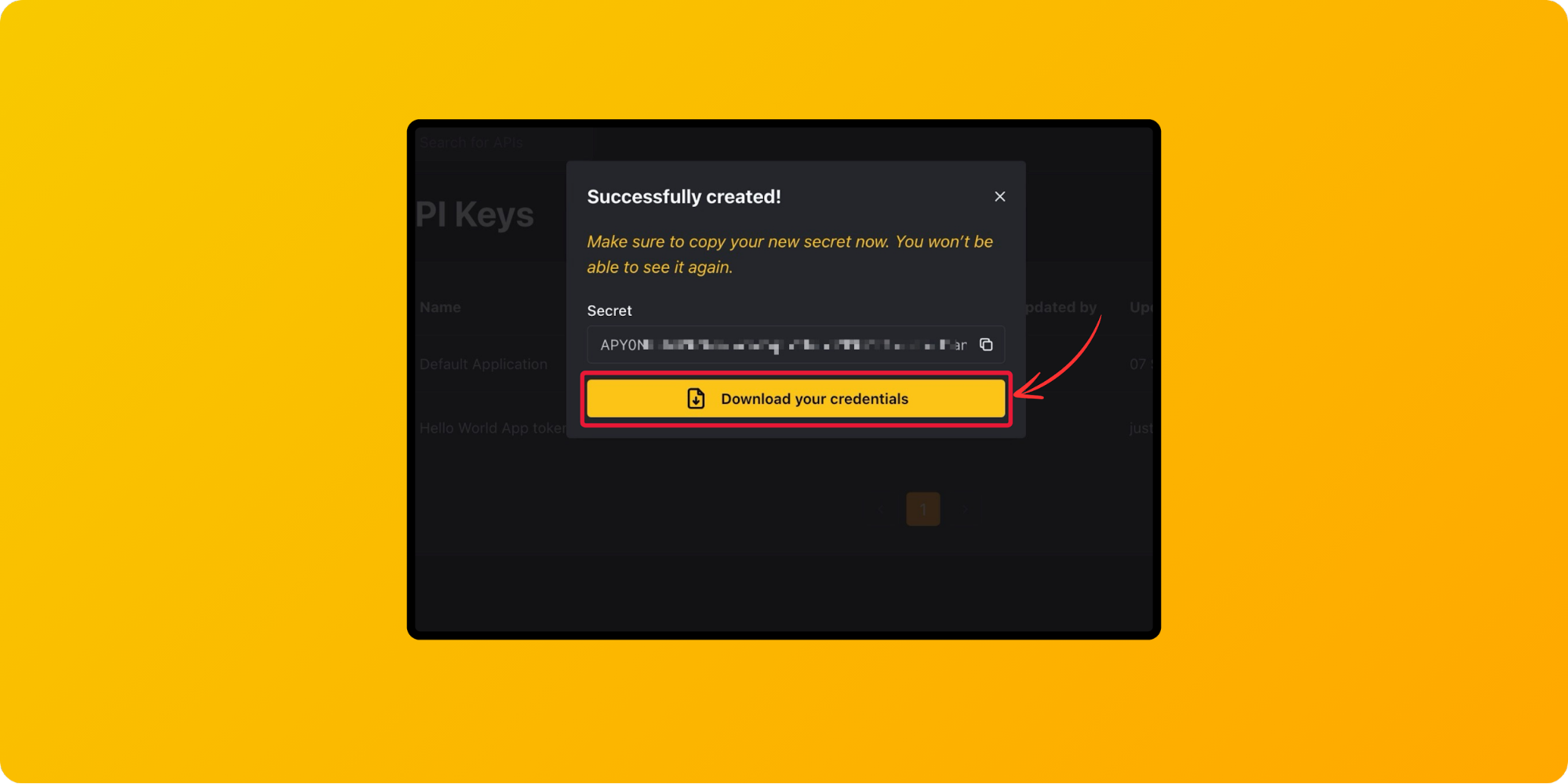
Security Considerations
Bearer tokens are secure but not bulletproof. Here’s what to watch:
- HTTPS Only: Unencrypted HTTP leaks tokens. Always enforce TLS.
- Storage: On clients, use secure vaults (e.g., Keychain,
localStorage
with sanitization). Never log tokens. - Expiration: Short-lived tokens (e.g., 15 minutes) limit exposure. Pair with refresh tokens.
- Revocation: OAuth-style revocation lists help kill compromised tokens. JWTs alone lack this—plan accordingly.
- Scope: Limit token privileges. A token for
/user
shouldn’t unlock/admin
.
ApyHub handles some of this, its tokens are scoped to specific APIs, reducing blast radius.
Tool Comparison in Real-Life Scenarios
Tools shape how bearer tokens perform. Let’s compare:
Postman
Postman’s a testing beast. You paste a token into the
Authorization
tab, hit send, and debug. For microservices, it’s great to chain requests to simulate token flows. But it’s manual. ApyHub automates this with prebuilt endpoints, cutting iteration time.AWS API Gateway
Gateway validates tokens against providers (e.g., Cognito). It’s robust for enterprise but config-heavy. ApyHub skips the setup—its catalog APIs are token-ready out of the gate.
Conclusion
Bearer token authentication nails the balance of security and simplicity. It’s stateless, signed, and versatile—perfect for APIs, microservices, and mobile apps. Challenges like token management exist, but tools like ApyHub smooth the edges. Its catalog of 130+ APIs leverages bearer tokens to slash boilerplate, letting you ship faster. Whether you’re validating emails or processing images, it’s a technical shortcut worth taking.
Want the shortcut? ApyHub’s catalog delivers 130+ battle-tested APIs. Need to process PDFs, validate data, handle payments—done.
FAQs About Bearer Token Authentication
Here are seven frequently asked questions to deepen your understanding of bearer token authentication and its practical use, including how tools like ApyHub streamline the process.
1. What is bearer token authentication, and why is it used?
Bearer token authentication secures APIs by passing a token (e.g., JWT) in the
Authorization
header. The server validates it to grant access without storing session data. It’s stateless, scalable, and secure, ideal for microservices and mobile apps. Using ApyHub, you can leverage bearer tokens with its 130+ APIs, skipping complex auth setup.2. How does a bearer token differ from an API key?
A bearer token, like a JWT, is dynamically signed, time-bound, and often scoped. API keys are static, lack expiration, and are less secure. Bearer tokens carry metadata (e.g., user ID, roles), making them versatile. ApyHub’s APIs use bearer tokens for secure, flexible access versus static key limitations.
3. Are bearer tokens safe to use in browsers?
Yes, but with care. Store tokens in memory or secure cookies, not
localStorage
, to avoid XSS risks. Use HTTPS to encrypt traffic. ApyHub mitigates client-side risks by scoping tokens to specific APIs, reducing exposure in single-page applications.4. How do I handle token expiration?
Tokens have an expiration (e.g.,
"exp": 1698777600
). When expired, servers reject them. Use refresh tokens to fetch new ones without re-authenticating. ApyHub’s APIs handle token lifecycle smoothly, issuing short-lived tokens and clear error codes for expiration.5. Can I revoke a bearer token?
Standard JWTs lack built-in revocation. You’d need a blacklist or OAuth-style revocation list. For critical apps, use short-lived tokens. ApyHub’s token system ties access to specific APIs, so revoking access is as simple as regenerating a token in its dashboard.
6. How does ApyHub simplify bearer token authentication?
ApyHub provides pre-built APIs that use bearer tokens out of the box. You grab a token from its dashboard and send it in the
Authorization
header, no auth server needed. For example:import requests
token = "YOUR_ApyHub_TOKEN"
headers = {"Authorization": f"Bearer {token}"}
response = requests.post("<https://api.ApyHub.com/validate/email>", headers=headers, json={"email": "test@example.com"})
This cuts setup time for tasks like data validation or PDF processing.
7. What happens if a bearer token is compromised?
A leaked token grants access until it expires. Short expiration times (e.g., 15 minutes) limit damage. Use scopes to restrict privileges. ApyHub’s APIs minimize risk by scoping tokens to specific endpoints, ensuring a stolen token can’t wreak havoc across unrelated services.