Engineering
What Are the Most Common APIs Used by Front-End Developers?
Discover essential APIs for frontend developers and how to integrate them effectively. Learn to optimize your workflow with ApyHub's ready-to-use development solutions.
SO
Sohail Pathan
Last updated on March 21, 2025
Need real-time weather data? Interactive maps? Secure payments? APIs turn these complex tasks into a few lines of code. No reinventing the wheel — just sleek, fast, user-focused apps.
Want the shortcut? ApyHub’s catalog delivers 130+ battle-tested APIs. Need to process PDFs, validate data, handle payments — done.
Let’s break down why APIs are your front-end superpower.
Why Should Developers Explore Third-Party APIs?
Third-party APIs are like cheat codes for front-end development. Here’s why you should jump on board:
Save Development Time: Why spend weeks building a feature when an API can do it in hours? Plug it in and move on to the fun stuff.
Access Advanced Features: Need AI-powered summaries or geolocation? Third-party APIs give you cutting-edge tools without requiring you to be an expert.
Reduce Costs: Building your own solutions can drain your budget. APIs often come with affordable pay-as-you-go plans, keeping your wallet happy.
14 Common APIs for Front-End Developers
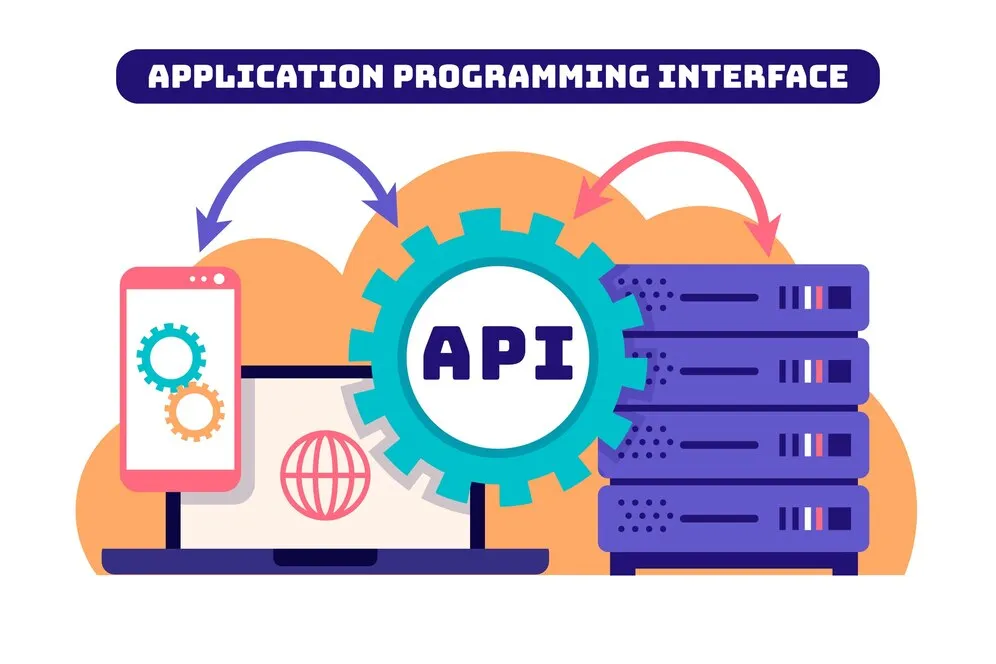
-
Currency Conversion API: Provides real-time exchange rates for global currencies and conversions.
-
Word to PDF API: Converts Word documents into universally compatible PDF files.
-
Extract Text from PDF API: Extracts text from PDFs, including OCR for scanned documents.
-
Extract Text from Webpage API: Scrapes text content from webpages, filtering out noise like ads.
-
AI Summarize API: Generates concise text summaries using AI technology.
-
SERP Rank Checker API: Tracks keyword rankings on search engines like Google or Bing.
-
ICal API: Manages calendar events in iCal format for scheduling integrations.
-
Weather API: Delivers current weather data and forecasts for any location.
-
Payment Gateway API (e.g., Stripe): Processes secure online payments and transactions.
-
Google Maps API: Embeds interactive maps and location-based services.
-
Twilio API: Enables SMS, voice, and messaging capabilities for communication.
-
YouTube Data API: Accesses YouTube video data for embedding or analysis.
-
Unsplash API: Supplies high-quality, royalty-free images for visual enhancements.
-
SendGrid API: Manages email sending, tracking, and deliverability.
These APIs are the backbone of countless applications, offering pre-built solutions to common development challenges. Let's dive into details.
1. Currency Conversion API
Overview: This API delivers real-time exchange rates for various currencies, often supporting historical data and cryptocurrency conversions. It’s a must-have for apps dealing with international transactions.
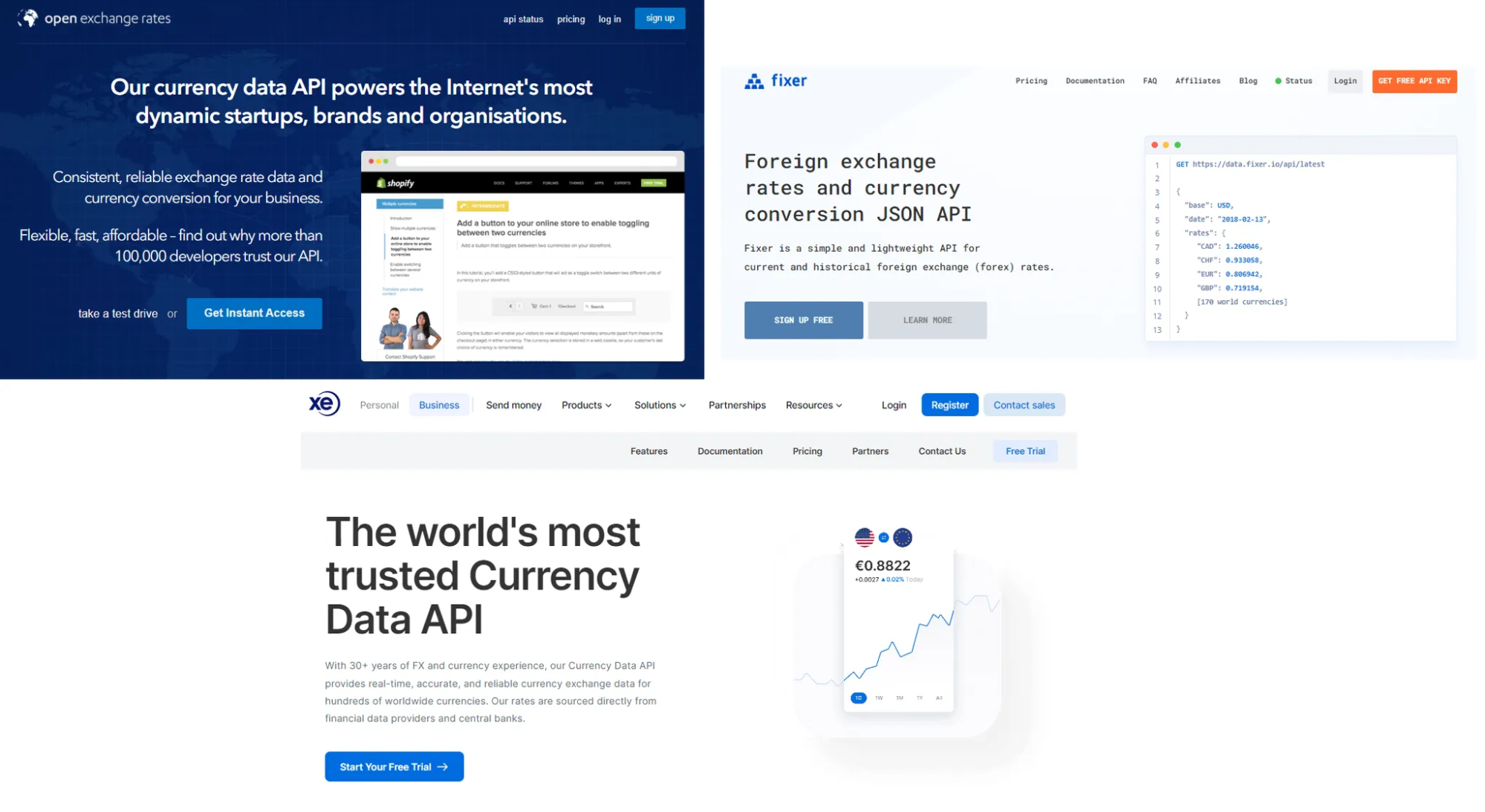
Key Features: Real-time rates, multi-currency support, historical data access.
Use Case: E-commerce platforms displaying prices in local currencies or travel apps calculating trip costs.
Implementation: Use a simple fetch request to retrieve and display converted amounts.
Code Example:
Note: Replace 'YOUR_API_KEY' with a key from providers like Open Exchange Rates.
2. Word to PDF API
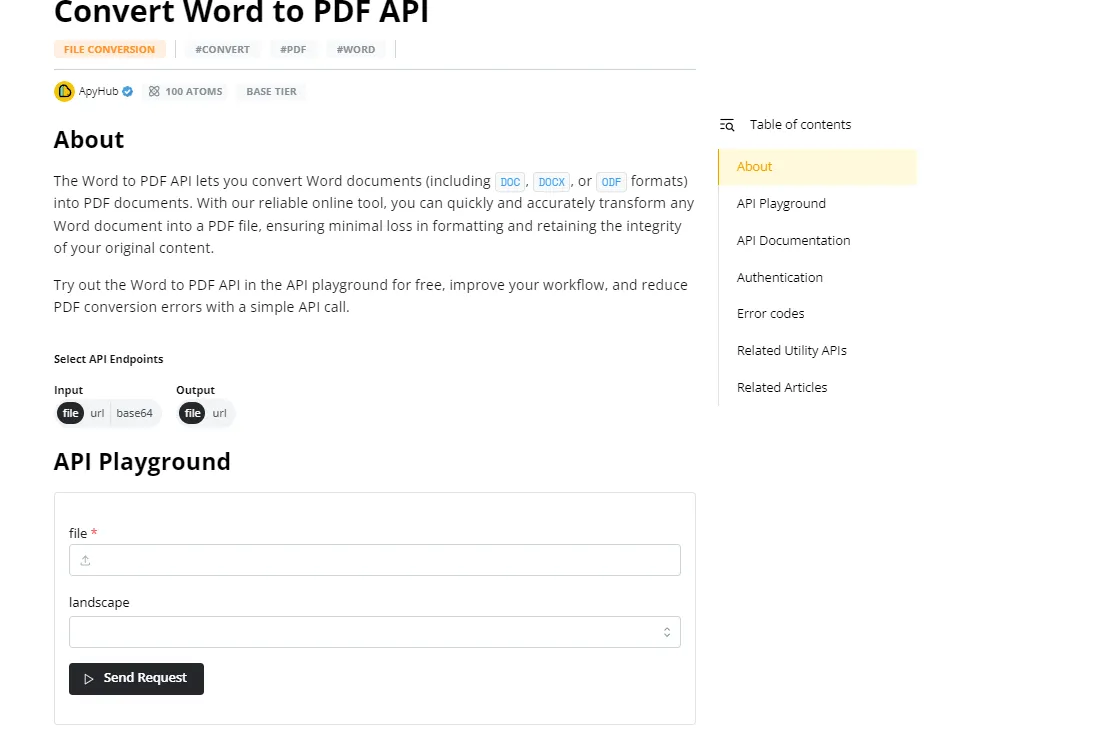
Overview: Converts Word documents (.docx, .doc) to PDF, preserving formatting for universal compatibility.
Key Features: Supports complex layouts, and batch processing for multiple files.
Use Case: Generating invoices or reports from user-uploaded files in an app.
Implementation: Upload a file via a form and download the converted PDF.
Code Example:
Note: Check Word to PDF Converter API by ApyHub.
3. Extract Text from PDF API
Overview: Extracts text from PDF files, including OCR for scanned documents, making static content usable.
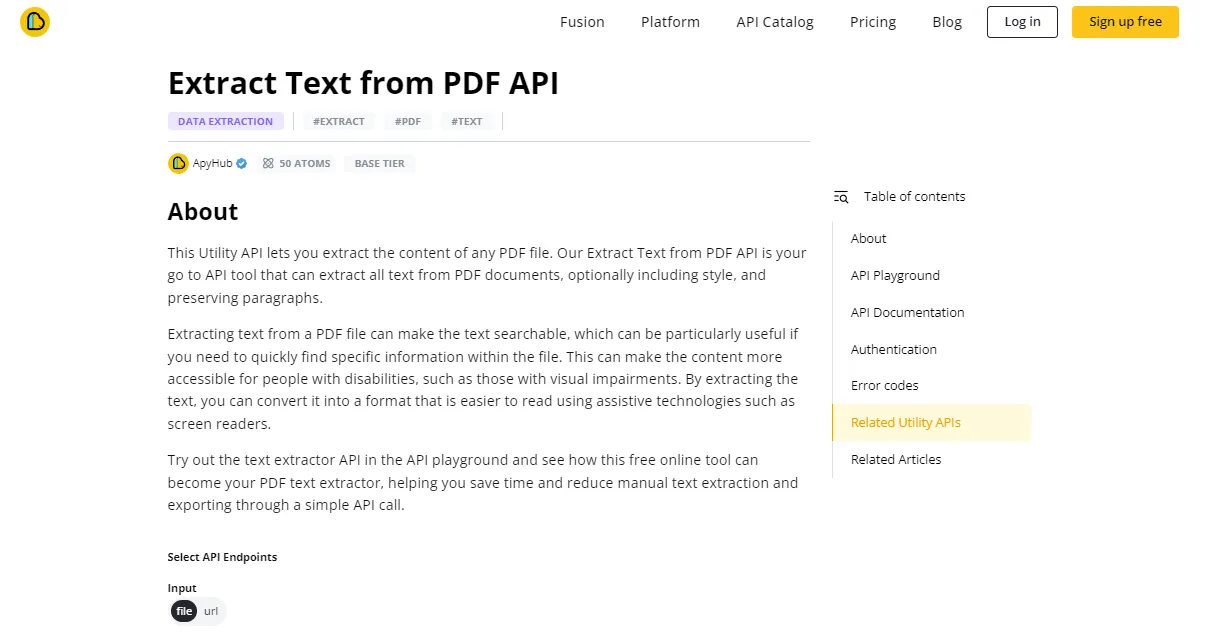
Key Features: OCR support, multi-page extraction.
Use Case: Digitizing resumes or invoices for data processing.
Implementation: Upload a PDF and display the extracted text.
Code Example:
Note: Enable OCR for scanned PDFs if supported.
4. Extract Text from Webpage API
Overview: Scrapes text from webpages, filtering out ads and navigation for clean content.
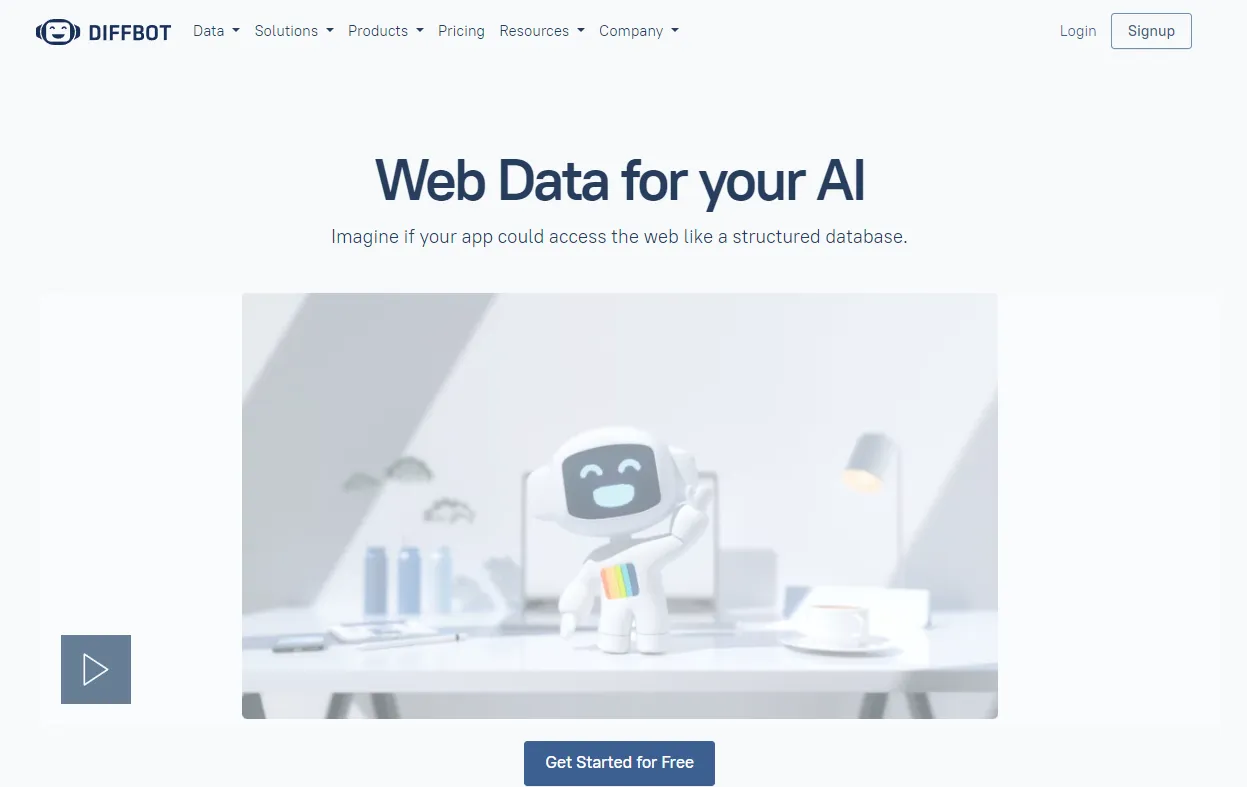
Key Features: Handles varied HTML, and supports dynamic sites.
Use Case: Building news aggregators or content dashboards.
Implementation: Fetch text from a URL and display it.
Code Example:
Note: Respect robots.txt policies.
5. AI Summarize API
Overview: Uses AI to create concise summaries of text, customizable by length or style.
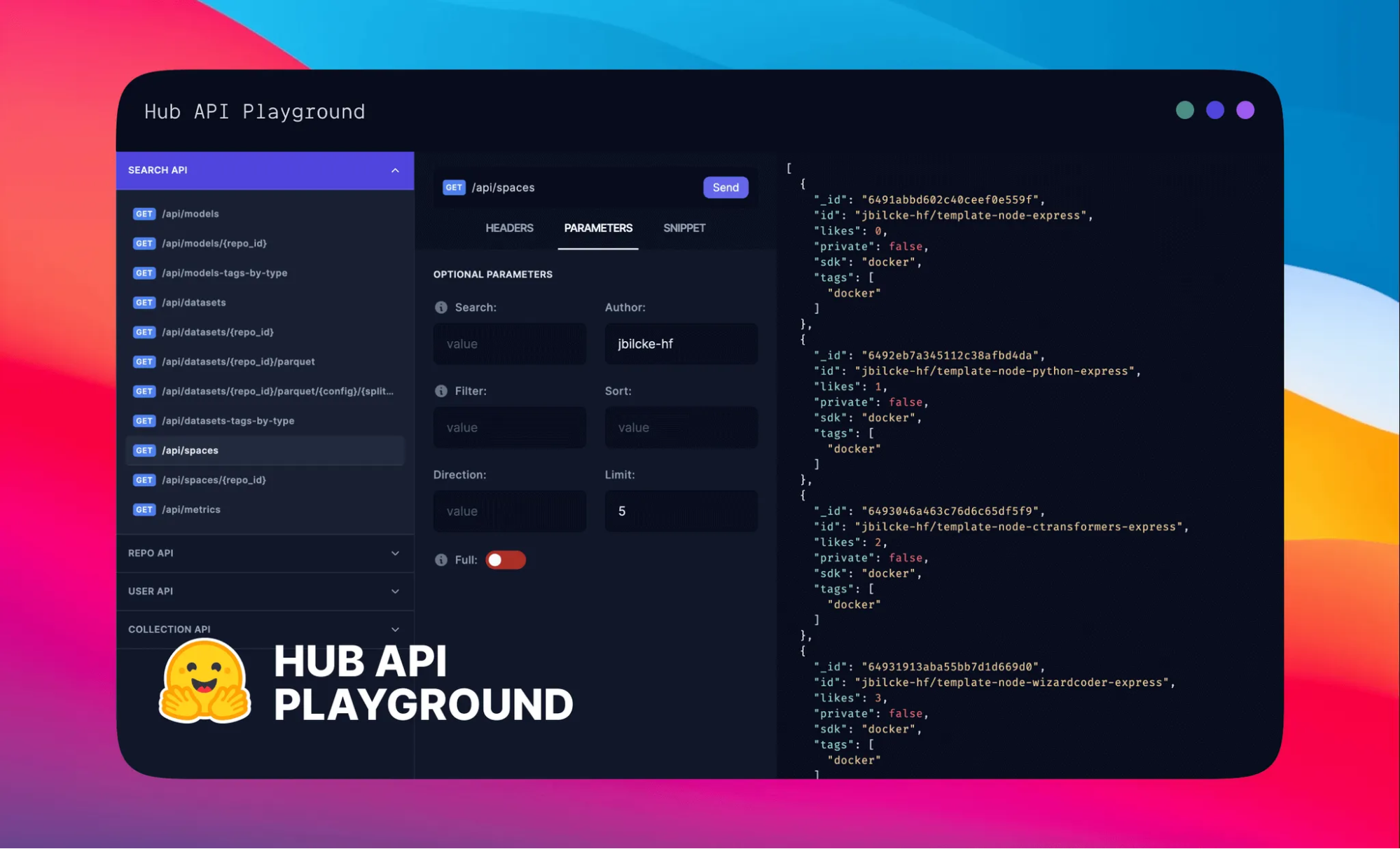
Key Features: Adjustable summary length, multiple output styles.
Use Case: Summarizing articles or reports for quick insights.
Implementation: Send text and display the summary.
Code Example:
6. SERP Rank Checker API
Overview: Monitors keyword rankings on search engines, providing SEO insights.
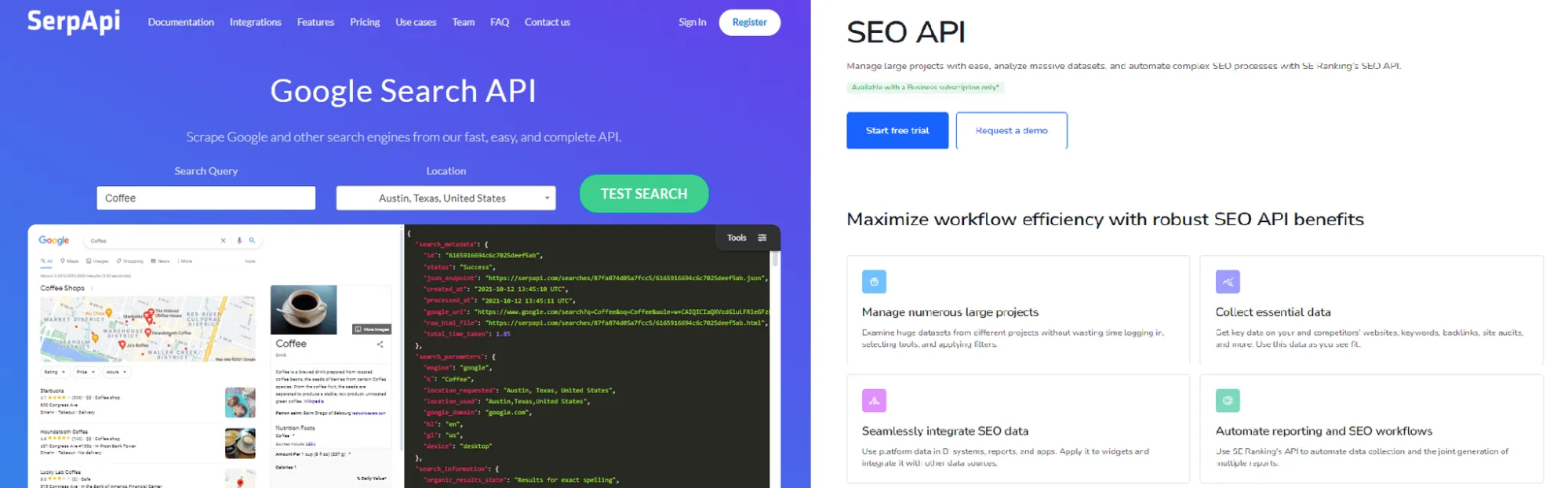
Key Features: Regional data, historical tracking.
Use Case: Optimizing site visibility for marketing campaigns.
Implementation: Check a keyword’s rank and display it.
Code Example:
7. ICal API
Overview: Creates and manages calendar events in iCal format for seamless syncing.
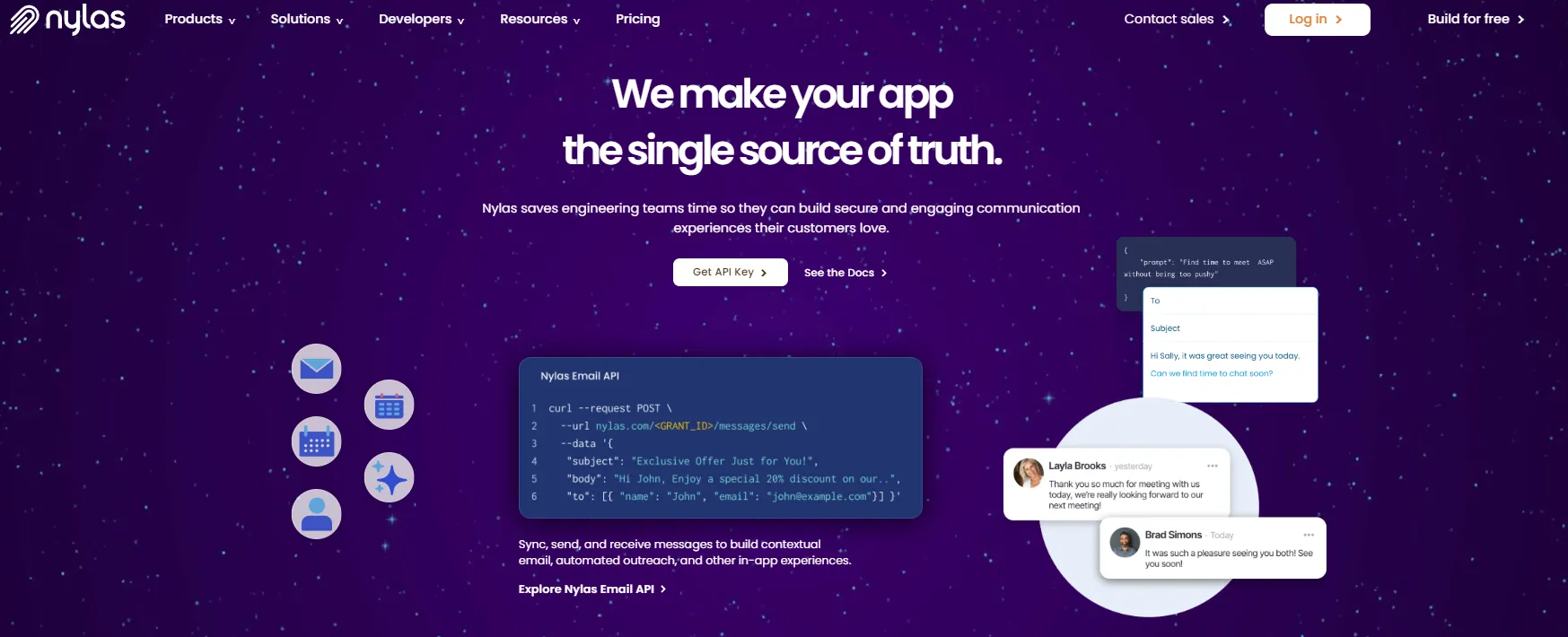
Key Features: Recurrence rules, time zone support.
Use Case: Scheduling appointments or events.
Implementation: Generate a downloadable .ics file.
Code Example:
8. Weather API
Overview: Provides current weather conditions and forecasts globally.
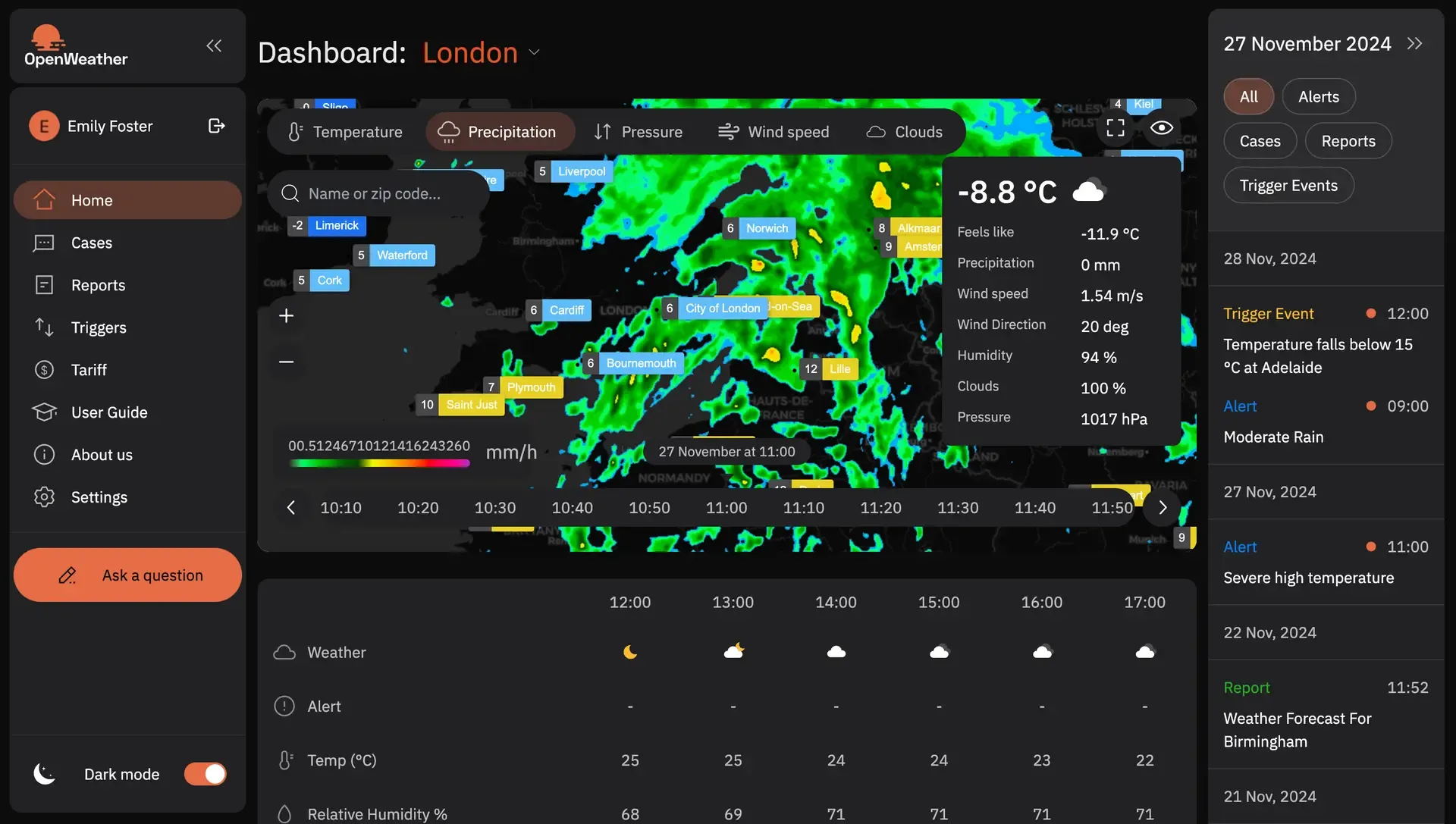
Key Features: Real-time data, multi-language support.
Use Case: Adding weather widgets to travel apps.
Implementation: Fetch and display weather data.
Code Example:
Note: Use an API key from OpenWeatherMap.
9. Payment Gateway API (e.g., Stripe)
Overview: Facilitates secure online payments with support for multiple methods.
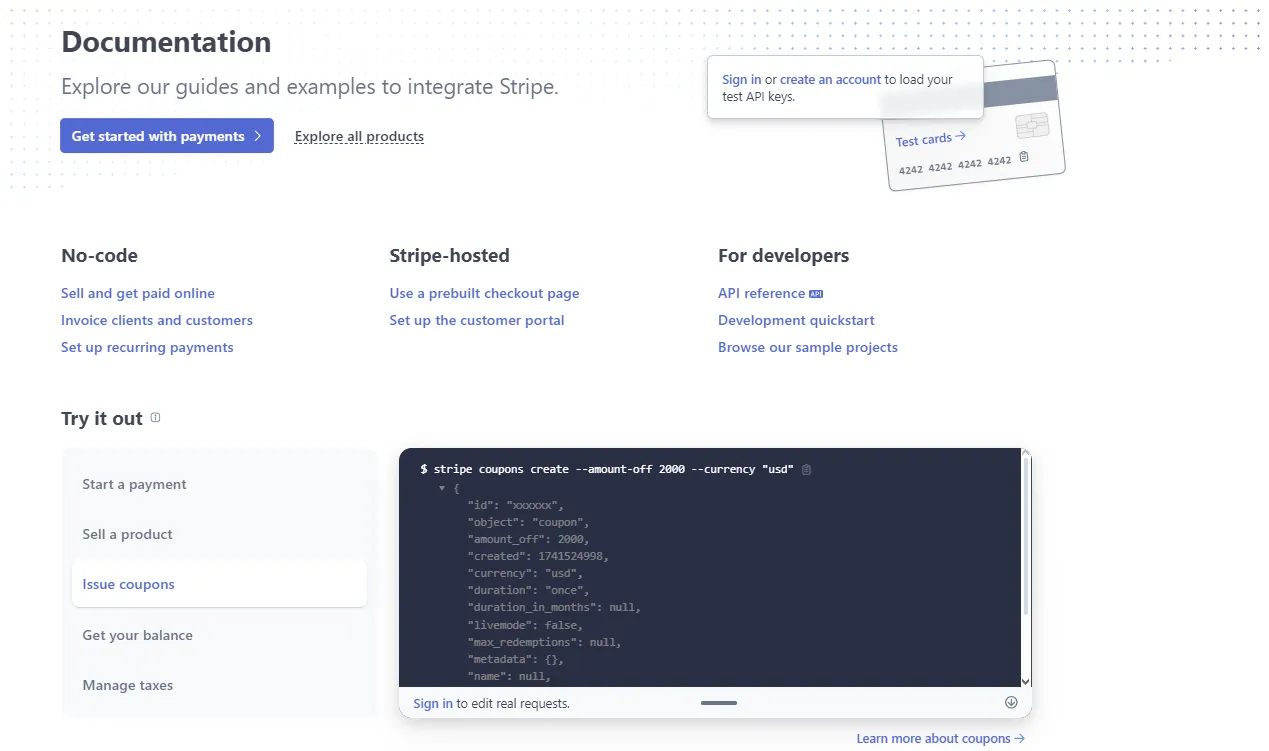
Key Features: Multi-currency support, fraud detection.
Use Case: E-commerce checkout systems.
Implementation: Set up a payment form with Stripe Elements.
Code Example:
Note: Requires server-side code for completion.
10. Google Maps API
Overview: Embeds interactive maps and location services into your app.
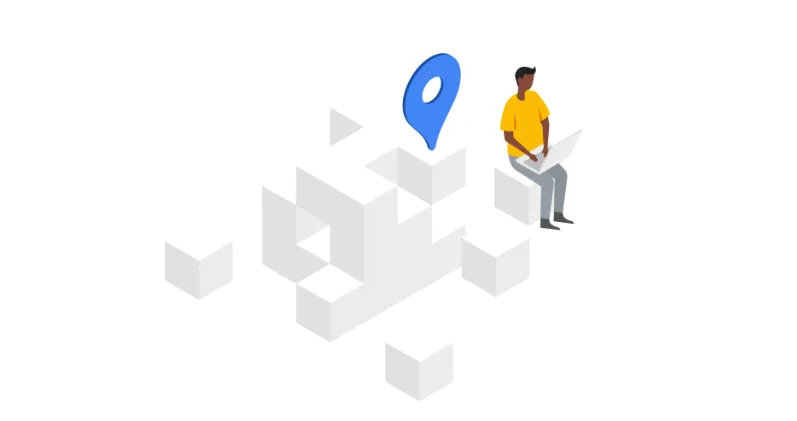
Key Features: Custom styling, real-time traffic data.
Use Case: Store locators or delivery tracking.
Implementation: Display a map with a marker.
Code Example:
Note: Get a key from Google Cloud Console.
11. Twilio API
Overview: Adds SMS, voice, and messaging capabilities to your app.
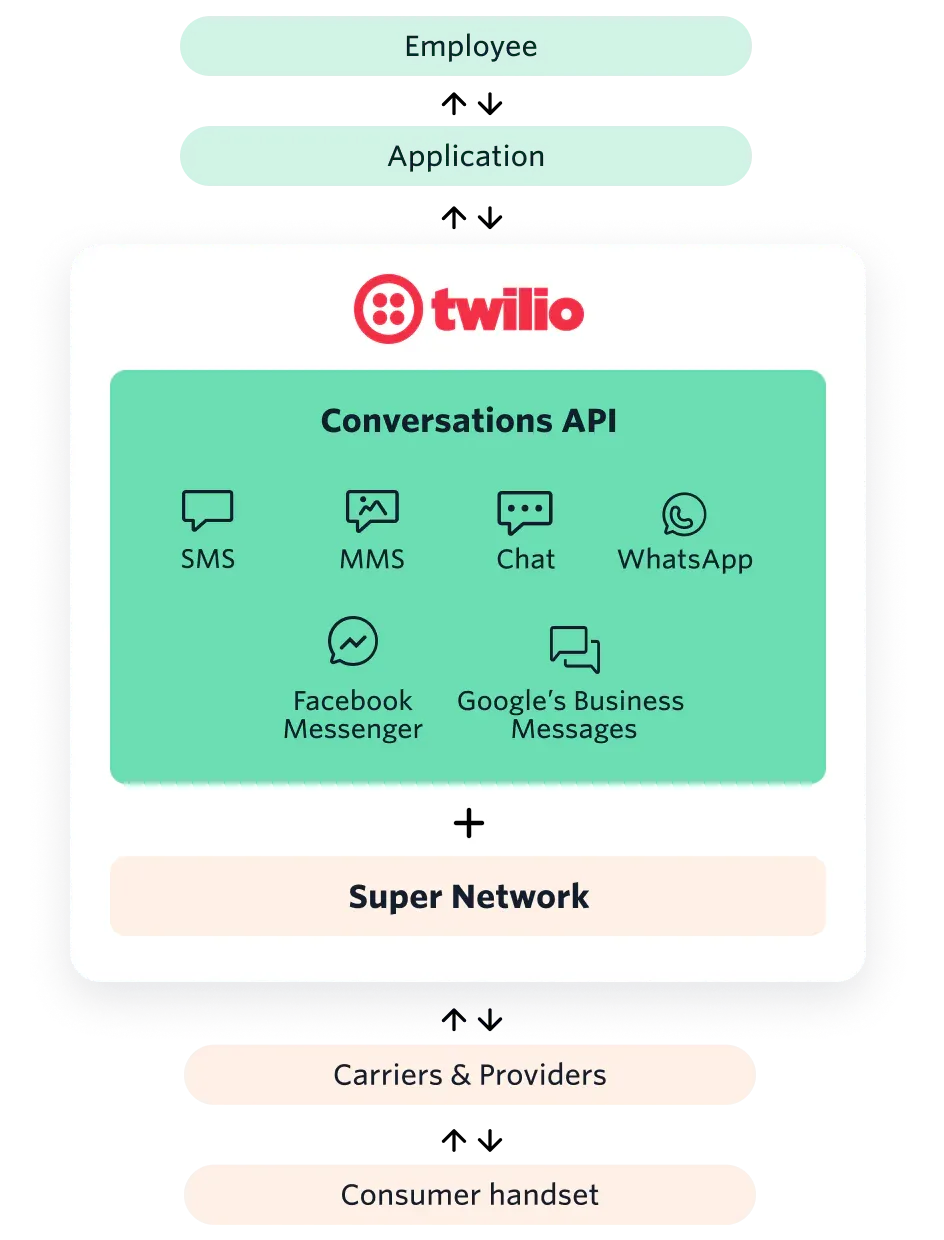
Key Features: Global reach, multi-channel support.
Use Case: Sending delivery notifications or 2FA codes.
Implementation: Send an SMS via server-side code.
Code Example (Node.js):
Note: Use Twilio credentials from their dashboard.
12. YouTube Data API
Overview: Accesses YouTube video data for integration or analysis.
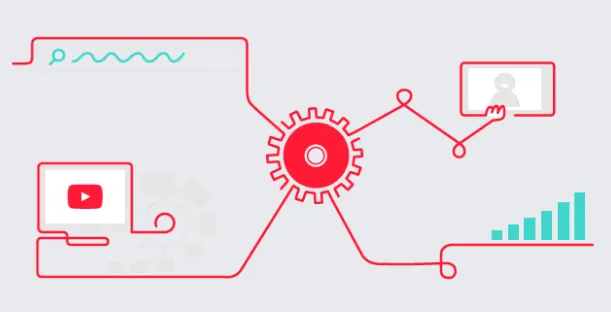
Key Features: Video search, playlist management.
Use Case: Building video galleries or tutorial hubs.
Implementation: Search and list video titles.
Code Example:
13. Unsplash API
Overview: Provides royalty-free, high-quality images for your app.
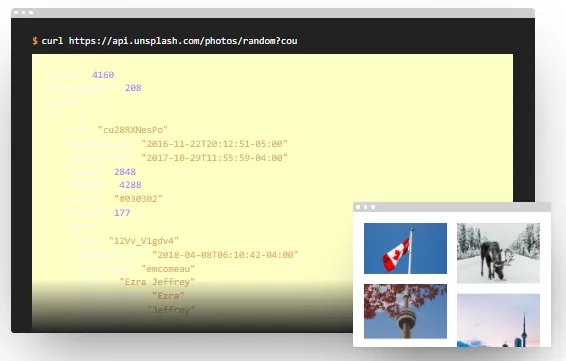
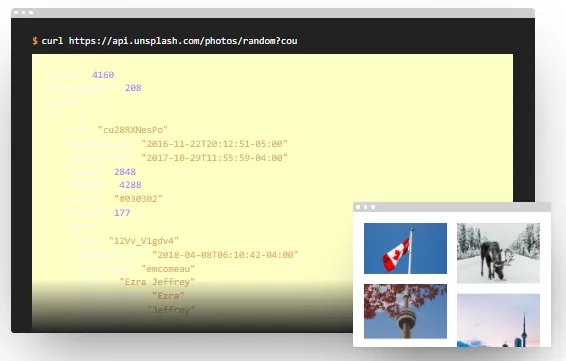
Key Features: Search by category, orientation filters.
Use Case: Enhancing UI with blog headers or backgrounds.
Implementation: Fetch and display a random photo.
Code Example:
Note: Get a key from Unsplash Developers.
14. SendGrid API
Overview: Manages email sending with tracking and deliverability features.
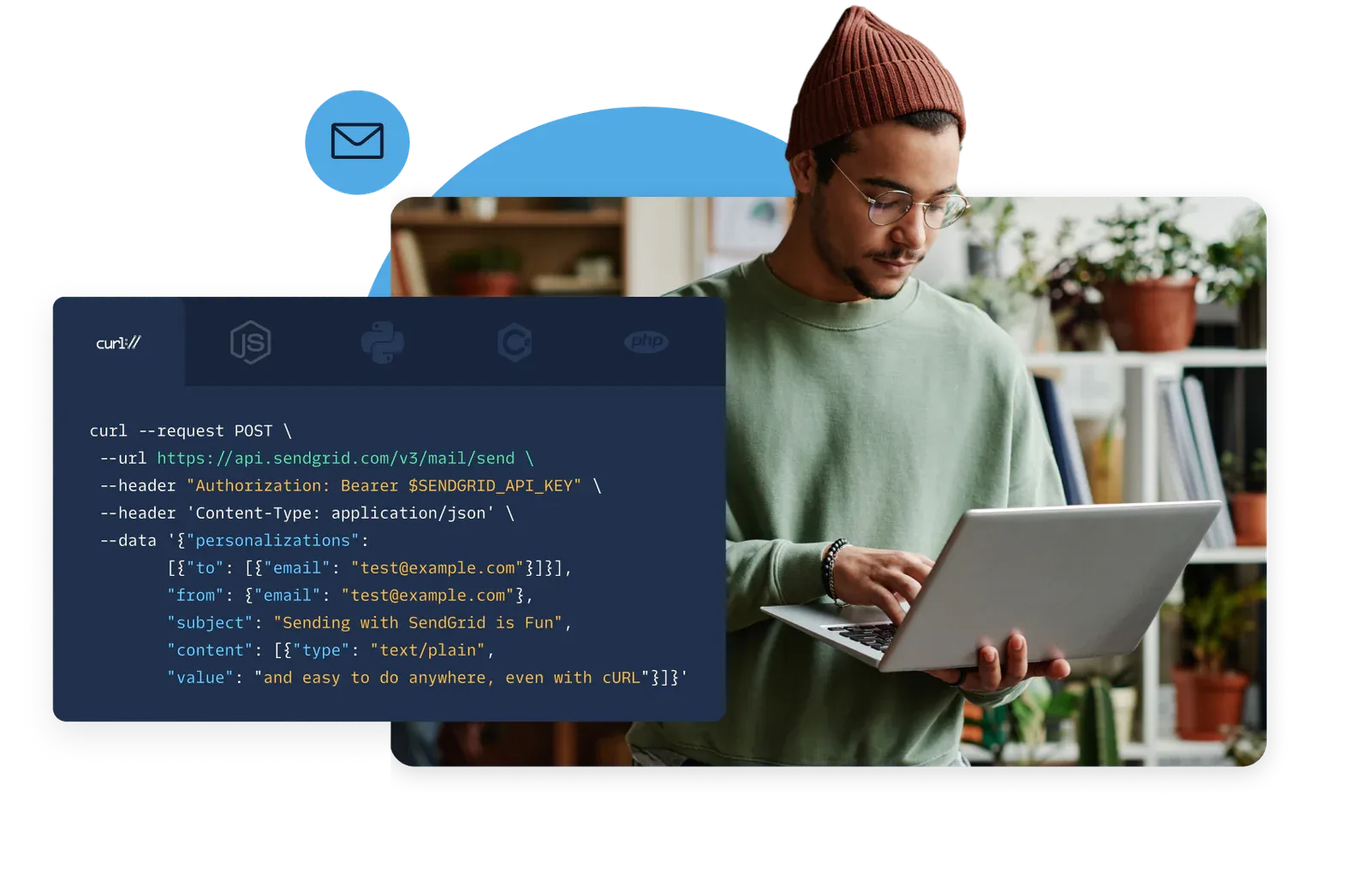
Key Features: Templates, analytics, bulk sending.
Use Case: Sending order confirmations or newsletters.
Implementation: Send an email via server-side code.
Code Example (Node.js):
Note: Verify your sender email with SendGrid.
Which File Formats Are Commonly Used by APIs and Web Services?
When an API hands over data, it’s usually wrapped in a specific file format. Here’s the lowdown on the most common ones:
JSON (JavaScript Object Notation): The king of API formats, JSON is lightweight, easy to read, and a breeze to parse in JavaScript. It’s the default choice for most modern APIs.
XML (eXtensible Markup Language): A bit old-school, XML is highly structured and great for complex data. But it’s bulky and trickier to work with than JSON.
CSV (Comma-Separated Values): Super simple and compact, CSV shines with tabular data like spreadsheets. However, it can’t handle nested structures.
Troubleshooting Common Issues with External APIs
APIs aren’t perfect, and things can go wrong. Here’s how to tackle the usual suspects:
CORS Errors: If you see a Cross-Origin Resource Sharing error, the API might not allow direct front-end requests. Check its CORS policy or use a backend proxy.
Rate Limits: Exceed the limit, and you’ll get blocked. Cache data or spread out requests to stay under the cap.
Auth Issues: Wrong API key? Misplaced header? Double-check the docs and your setup.
Bad Data: If the response breaks your app, log it and add fallback logic to handle surprises.
With a little debugging, you’ll resolve most issues and keep your app humming along.
Conclusion
These 14 APIs are your frontline allies for building sleek, user-friendly front-end apps quickly. They handle everything from file conversions to secure payments, freeing you to focus on crafting great user experiences.
For even more ready-to-use solutions, check out ApyHub’s extensive API catalog and elevate your projects today. Happy building!
FAQ
How do front-end developers handle API rate limiting in user-facing applications?
Implement client-side caching with localStorage or sessionStorage to reduce repeated calls. Use loading states and graceful degradation when limits are reached. For high-traffic apps, consider proxy services or ApyHub's scalable APIs with higher threshold limits.
What tools help test API integrations during front-end development?
Popular options include Postman for endpoint testing, Chrome DevTools for network inspection, and libraries like Axios Mock Adapter for simulating API responses during development.
Can front-end APIs work offline or in low-connectivity scenarios?
While most require the internet, developers can use service workers with Cache API to store critical API responses for offline access. Some data processing APIs (like text conversion) might need full connectivity.
How do modern front-end frameworks like React handle API integration differently?
Frameworks provide hooks (React Query, SWR) that optimize API calls with features like automatic revalidation, request deduping, and error retries, improving both performance and developer experience.
What’s the environmental impact of heavy API usage in web apps?
Each API call consumes energy. Developers can reduce impact by optimizing request frequency, using efficient data formats, and choosing green hosting providers. Some API providers now offer carbon-neutral solutions.