Engineering
How to Use REST Assured for Advanced API Testing
Master REST Assured for API testing with advanced techniques like JSON validation, authentication, and performance testing. Compare REST Assured, Postman, and ApyHub in real-world scenarios.
MU
Muskan Sidana
Last updated on April 17, 2025
APIs are the backbone of modern applications, and testing them isn’t optional it’s a requirement. REST Assured, a robust Java library, simplifies API testing automation with a clean, readable syntax designed to tackle RESTful endpoints efficiently.
Looking to streamline your API workflow even further? Check out ApyHub, a platform offering over 130+ production-ready APIs for tasks like PDF automation, data validation, and image processing. With ApyHub, you can skip the boilerplate and ship faster.
Let’s get into the code, workflows, and details you need to master REST Assured.
What is REST Assured?
REST Assured is a Java-based Domain-Specific Language (DSL) crafted for automating API testing with an emphasis on simplicity and readability. It streamlines the process of testing RESTful APIs by abstracting complex HTTP interactions into an intuitive, chainable syntax. Built on Java, it integrates seamlessly with the JVM ecosystem, making it a natural fit for teams already leveraging Java in their development or testing stacks.
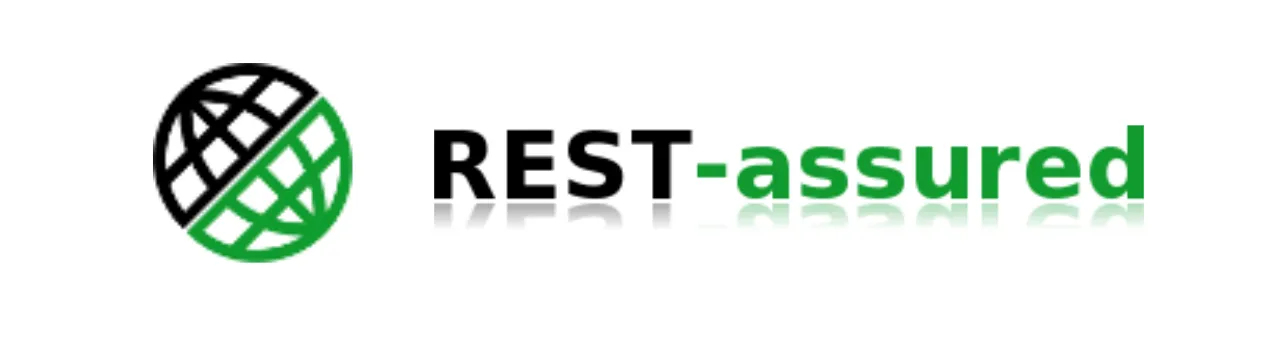
Key Features
- Support for HTTP Methods: Test GET, POST, PUT, DELETE, and more with a consistent, unified interface.
- **JSON and XML Validation**: Natively parse and validate API responses using JSONPath and XmlPath to ensure data accuracy.
- BDD-Style Syntax: Write readable, maintainable tests using a Behavior-Driven Development (BDD) approach with
given()
,when()
, andthen()
methods.
These features make REST Assured a go-to tool for engineers who need to validate APIs efficiently with minimal setup.
Why Choose REST Assured?
REST Assured earns its place as a top choice for API testing automation by delivering practical benefits that align with modern development workflows. Here’s why it stands out:
Advantages for Automation
- CI/CD Integration: As a Java library, it plugs effortlessly into Continuous Integration and Continuous Deployment (CI/CD) pipelines like Jenkins or GitHub Actions, enabling automated testing with every build.
- Code Reusability: With Java’s object-oriented design, you can build modular, reusable test components, reducing redundancy and easing maintenance.
- Scalability: From a single endpoint to a sprawling API suite, REST Assured handles large-scale testing without sacrificing performance.
These strengths make REST Assured a reliable ally in agile, fast-moving development environments where precision and speed are non-negotiable.
Prerequisites & Setup
To harness REST Assured effectively, you’ll need a solid foundation of tools and knowledge. This section covers the essentials and walks you through the setup process to kickstart your project.
Tools and Knowledge Needed
- Java 8+: Install a Java Development Kit (JDK) version 8 or higher REST Assured’s minimum requirement.
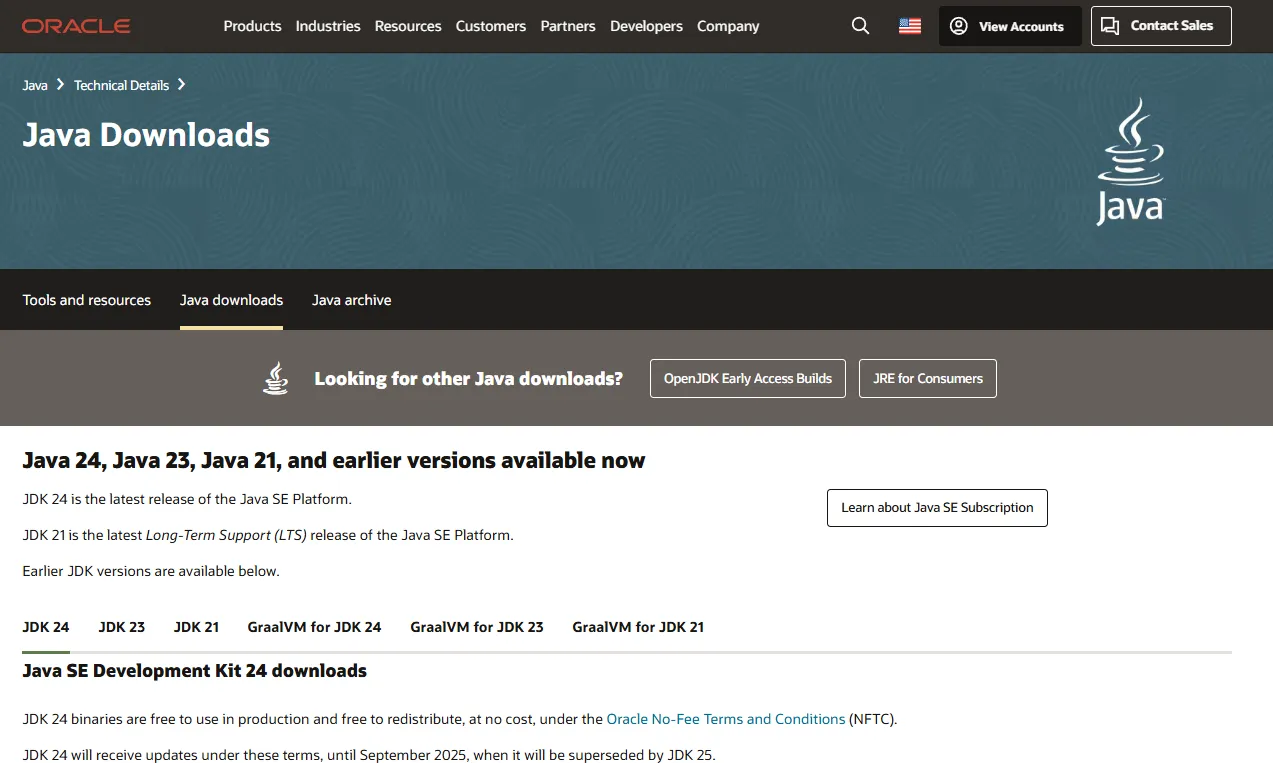
- IDE: Use an Integrated Development Environment like IntelliJ IDEA or Eclipse for a smoother coding and testing experience.
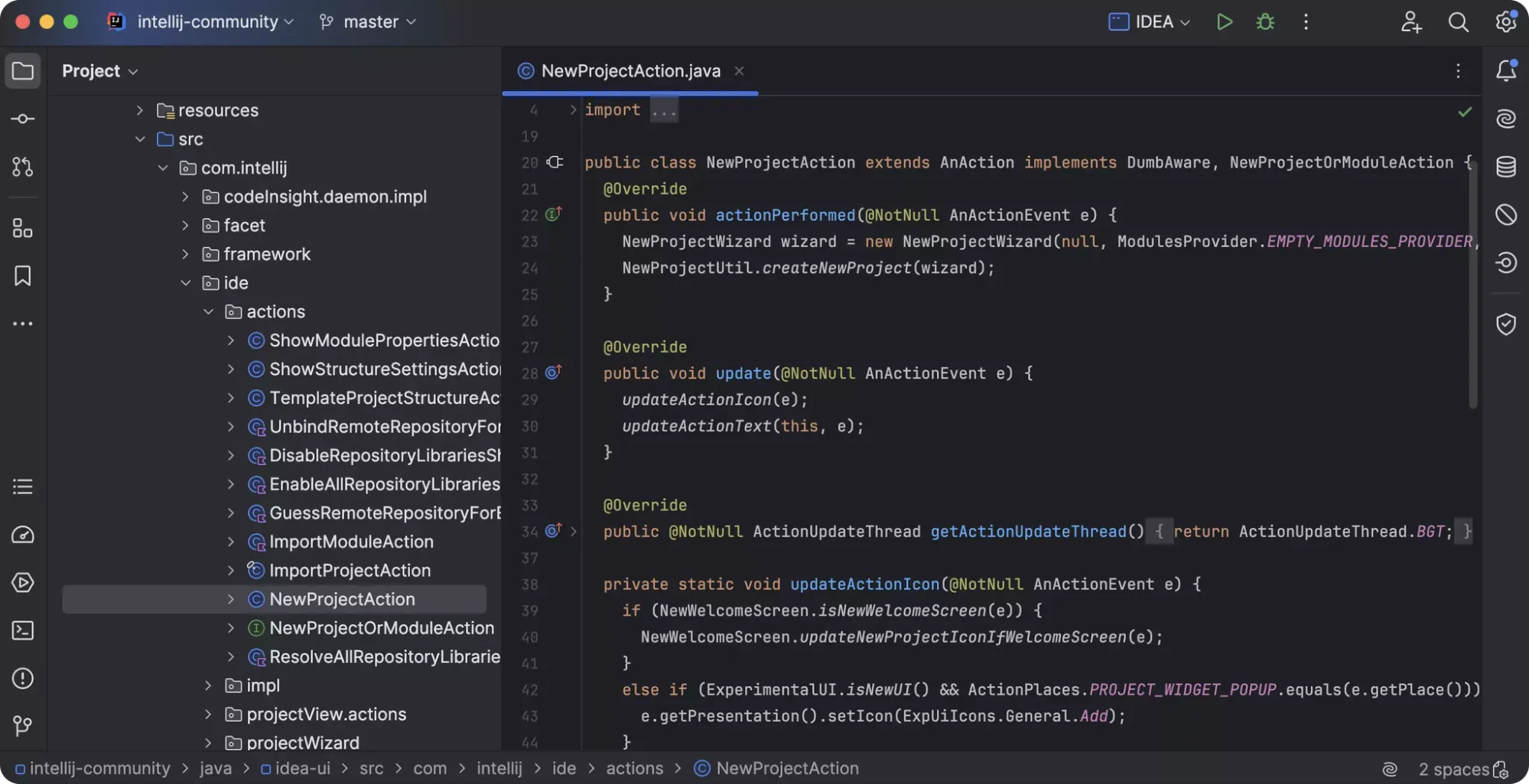
- Maven or Gradle: Manage dependencies with Maven or Gradle, the leading build tools for Java projects.
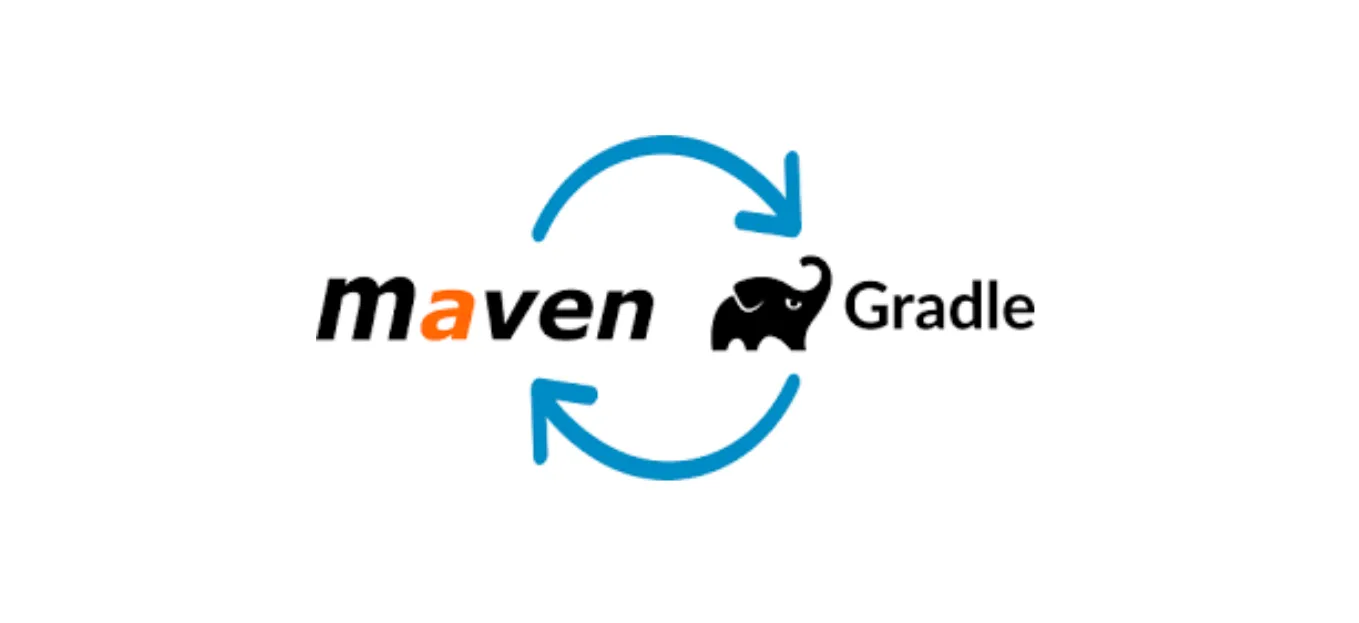
- Basic Understanding of REST APIs: Know your HTTP methods (GET, POST), status codes (200, 404), and payload formats (JSON/XML).
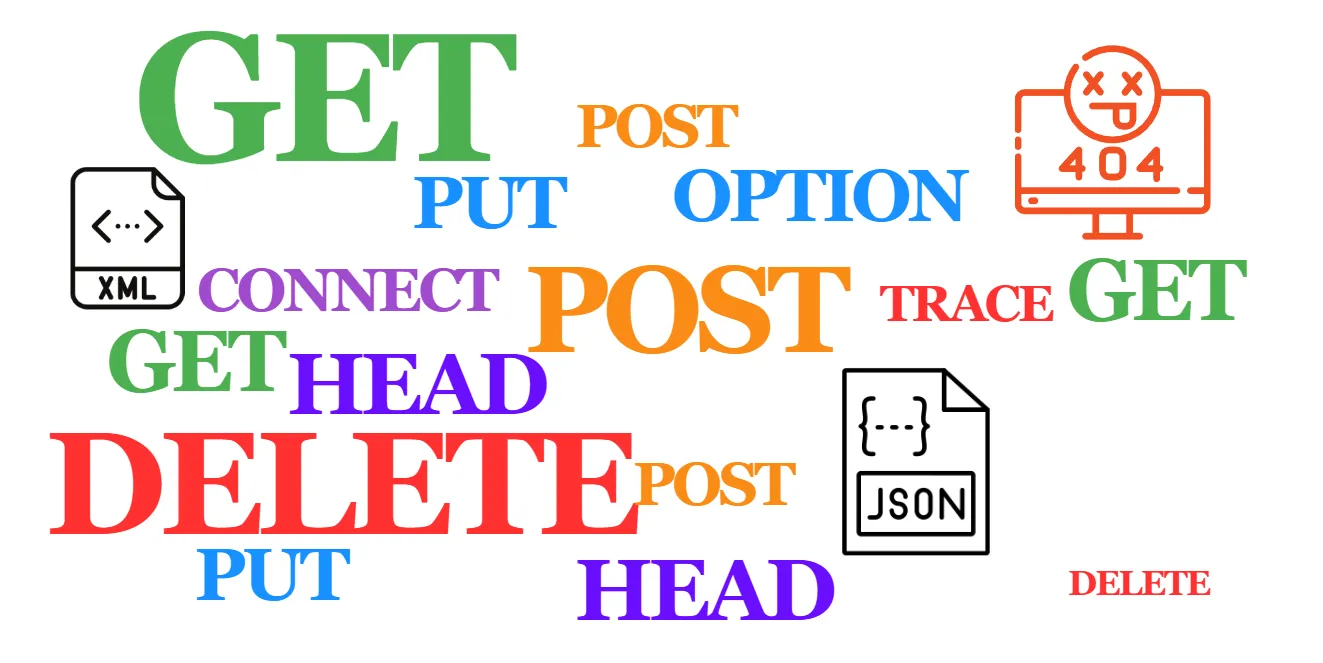
- Core Java Knowledge: Familiarity with Java basics classes, methods, and syntax is essential for writing effective tests.
Adding REST Assured to Your Project
Add REST Assured to your project by including its dependency in your build file. Below are the configurations for Maven and Gradle:
Maven
<dependency>
<groupId>io.rest-assured</groupId>
<artifactId>rest-assured</artifactId>
<version>5.5.0</version>
<scope>test</scope>
</dependency>
Gradle
testImplementation 'io.rest-assured:rest-assured:5.5.0'
For a testing framework like JUnit 5, add this dependency too:
Maven
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>5.10.0</version>
<scope>test</scope>
</dependency>
Gradle
testImplementation 'org.junit.jupiter:junit-jupiter-engine:5.10.0'
Base URI Configuration for Test Suites
Set a base URI to keep your tests clean and avoid repeating the API’s root URL. Here’s how to configure it in a setup method:
import io.restassured.RestAssured;
import org.junit.jupiter.api.BeforeAll;
public class BaseTest {
@BeforeAll
public static void setup() {
RestAssured.baseURI = "<https://jsonplaceholder.typicode.com>";
}
}
With this, your tests can use relative paths (e.g.,
/users
) instead of full URLs, adhering to the DRY (Don’t Repeat Yourself) principle.Writing Hard-Hitting API Tests
REST Assured’s BDD-style DSL gets straight to the point. Here’s a GET request test against JSONPlaceholder:
import static io.restassured.RestAssured.*;
import static org.hamcrest.Matchers.*;
import org.junit.jupiter.api.Test;
public class QuickGetTest {
@Test
public void validateUsersEndpoint() {
given()
.when()
.get("/users")
.then()
.statusCode(200)
.body("[0].name", equalTo("Leanne Graham"))
.header("Content-Type", containsString("application/json"));
}
}
This test validates the status code, response body, and headers in a concise chain pure efficiency.
Advanced Techniques for REST Assured
Let’s explore REST Assured’s advanced capabilities to tackle real-world API challenges.
Mastering HTTP Methods
Beyond GET, test a POST request to create a resource:
import io.restassured.http.ContentType;
import static io.restassured.RestAssured.*;
import org.junit.jupiter.api.Test;
public class PostResourceTest {
@Test
public void createNewUser() {
String payload = "{ \\\\"name\\\\": \\\\"Jane Doe\\\\", \\\\"email\\\\": \\\\"jane@example.com\\\\" }";
given()
.contentType(ContentType.JSON)
.body(payload)
.when()
.post("/users")
.then()
.statusCode(201)
.body("name",equalTo("Jane Doe"));
}
}
For DELETE or PUT, adjust the method and assertions REST Assured keeps the syntax consistent.
Parsing JSON and XML Like a Pro
Handle complex responses with JSONPath:
import io.restassured.response.Response;
import static io.restassured.RestAssured.*;
import org.junit.jupiter.api.Test;
public class ParseResponseTest {
@Test
public void extractNestedData() {
Response response = given()
.when()
.get("/users/1")
.then()
.extract().response();
String companyName = response.jsonPath().getString("company.name");
assert companyName.equals("Romaguera-Crona");
}
}
For XML, use
.xmlPath().getString("root.element")
. REST Assured supports both formats natively.Handling Authentication
Secure APIs often require authentication. Here’s an OAuth2 example:
import static io.restassured.RestAssured.\*;
import org.junit.jupiter.api.Test;
public class OAuthTest {
@Test
public void testSecuredEndpoint() {
String token = "your-oauth2-token-here";
given()
.header("Authorization", "Bearer"+ token)
.when()
.get("/secure-data")
.then()
.statusCode(200);
}
}
For Basic Auth, use
.auth().basic("user", "pass")
. REST Assured adapts effortlessly.Parameterizing for Scale
Avoid hardcoding with JUnit 5’s
@CsvSource
:import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.CsvSource;
import static io.restassured.RestAssured.*;
public class ParameterizedTest {
@ParameterizedTest
@CsvSource({
"1, Leanne Graham",
"2, Ervin Howell"
})
public void testMultipleUsers(int id, String name) {
given()
.pathParam("id", id)
.when()
.get("/users/{id}")
.then()
.body("name",equalTo(name));
}
}
This approach scales your tests efficiently, pulling data from various sources as needed.
Best Practices to Keep Tests Sharp
- Group by Endpoint: Organize tests by resource (e.g.,
/users
,/posts
). - Assert Smart: Focus on critical fields and status codes avoid brittle tests.
- Log Everything: Use
.log().all()
ingiven()
andthen()
for debugging. - CI/CD Integration: Run tests in Jenkins or GitHub Actions to catch issues early.
- Clean Up: Delete resources created during tests to maintain a tidy environment.
Real-World Use Cases: REST Assured vs. Others
Here’s how REST Assured stacks up against tools like Postman and SoapUI in practical scenarios.
Case 1: Payment Gateway API
Test a secure payment API with OAuth2:
@Test
public void testPaymentCreation() {
String token = getOAuthToken(); // Helper method assumed
String payload = "{\\\\"amount\\\\": 100.00, \\\\"currency\\\\": \\\\"USD\\\\", \\\\"card\\\\": \\\\"4111-1111-1111-1111\\\\"}";
given()
.header("Authorization","Bearer"+ token)
.contentType(ContentType.JSON)
.body(payload)
.when()
.post("/transactions")
.then()
.statusCode(201)
.body("status", equalTo("processed"));
}
Postman: Fine for manual tests, but automation requires scripting hacks. REST Assured wins for CI/CD integration.
Case 2: Nested E-Commerce API
Validate a complex JSON response:
@Test
public void testProductDetails() {
given()
.when()
.get("/products/123")
.then()
.body("variants[0].price", equalTo(29.99f))
.body("variants.findAll { it.inStock }.size()", greaterThan(0));
}
SoapUI: Functional but clunky with its GUI. REST Assured’s code-first approach is faster and version-controlled.
Case 3: WebSocket Fallback API
Test a REST fallback for a chat app:
@Test
public void testChatFallback() {
given()
.queryParam("userId", "abc123")
.when()
.get("/messages")
.then()
.statusCode(200)
.body("messages", hasSize(greaterThan(0)));
}
Custom Scripts: Python works, but REST Assured ties into Java ecosystems more tightly.
What We Like and Don’t Like About REST Assured
What We Like
- Readable DSL:
given().when().then()
chains are intuitive and concise. - Framework Fit: Integrates seamlessly with JUnit, TestNG, and CI/CD tools.
- Response Parsing: JSONPath and XmlPath make data extraction precise.
- Community Support: Strong documentation and an active user base.
What We Don’t Like
- Java-Only: No support for Python or JavaScript JVM exclusive.
- Verbose Debugging: Logging helps, but large responses can be tricky to troubleshoot.
- No GUI: Lacks Postman’s visual ease for ad-hoc testing.
Conclusion
REST Assured empowers testers to tackle complex API scenarios with precision, from nested JSON validation to performance testing. Its programmatic approach outperforms GUI-based tools like Postman in automation-heavy workflows, while its flexibility complements platforms like ApyHub. Whether you’re handling authentication, file operations, or schema validation, REST Assured delivers robust solutions with minimal overhead.
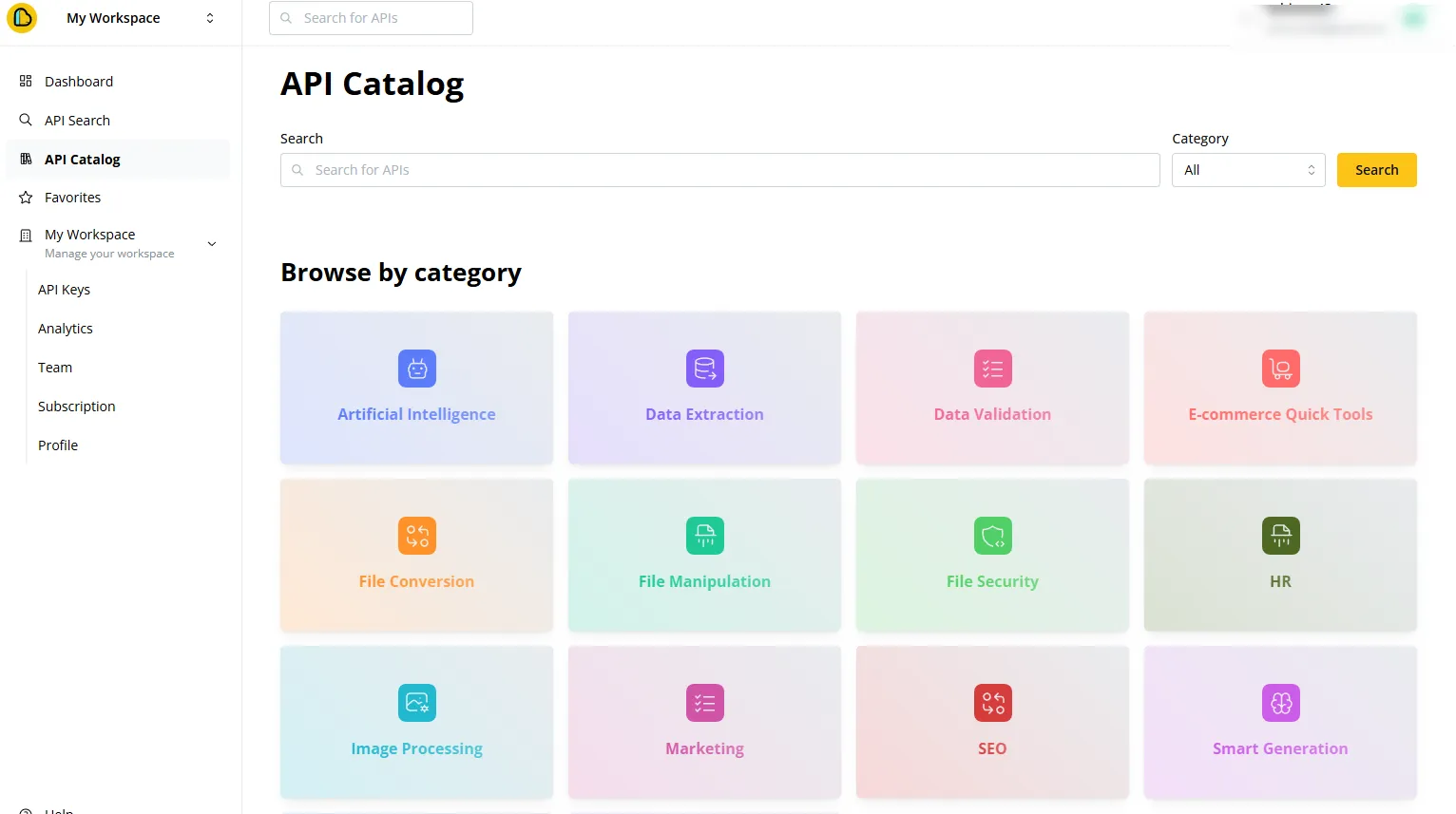
Ready to simplify your API testing? ApyHub’s catalog of 130+ APIs covering PDF automation, data validation, and more integrates seamlessly with REST Assured. Test ApyHub’s endpoints to validate data or process files without writing extra code. Explore ApyHub today and ship faster.
FAQs About REST Assured and API Testing
Below are answers to common questions about REST Assured, API testing, and how tools like ApyHub can enhance your workflow.
1. What makes REST Assured different from other API testing tools?
REST Assured stands out with its Java-based DSL, offering a BDD-style syntax that’s both readable and powerful. Unlike GUI-driven tools like Postman, it’s code-first, making it ideal for automation and CI/CD integration. Its native support for JSON/XML parsing and HTTP methods ensures precision.
2. Can I use REST Assured for testing non-REST APIs?
Yes, REST Assured is flexible enough to test any HTTP-based API, including non-REST endpoints like SOAP or GraphQL. For SOAP, use its XML parsing capabilities; for GraphQL, handle POST requests with query payloads. If you’re testing diverse APIs, ApyHub offers endpoints for varied tasks, data validation, file conversion that you can validate with REST Assured’s versatile syntax.
3. How do I handle dynamic API responses in REST Assured?
Dynamic responses (e.g., random IDs, timestamps) are common. Use JSONPath or Hamcrest matchers to focus on stable fields. For example:
.then().body("data.id", notNullValue()).body("data.name", equalTo("Expected Name"));
4. Is REST Assured suitable for large-scale API testing projects?
Absolutely. REST Assured scales well with parameterized tests, reusable methods, and CI/CD integration. Organize tests by endpoint and use data-driven approaches (e.g., JUnit’s
@CsvSource
).5. How can I debug failing REST Assured tests effectively?
Enable detailed logging with
.log().all()
in given()
and then()
to capture requests and responses. Check status codes, headers, and body content systematically. If parsing fails, verify JSONPath/XPath expressions. When testing ApyHub APIs, like PDF generation, use REST Assured’s logging to inspect payloads and ensure inputs align with ApyHub’s documented formats, making debugging faster.